LibreOffice Developer's Guide: Chapter 7 - Text Documents
In the LibreOffice API, a text document is a document model which is able to handle text contents. A document in our context is a product of work that can be stored and printed to make the result of the work a permanent resource. By model, we mean data that forms the basis of a document and is organized in a manner that allows working with the data independently of their visual representation in a graphical user interface.
It is important to understand that developers have to work with the model directly, when they want to change it through the LibreOffice API. The model has a controller object which enables developers to manipulate the visual presentation of the document in the user interface. But the controller is not used to change a document. The controller serves two purposes.
- The controller interacts with the user interface for movement, such as moving the visible text cursor, flipping through screen pages or changing the zoom factor.
- The second purpose is getting information about the current view status, such as the current selection, the current page, the total page count or the line count. Automatic page or line breaks are not really part of the document data, but rather something that is needed in a certain presentation of the document.
Keeping the difference between model and controller in mind, we will now discuss the parts of a text document model in the LibreOffice API.
The text document model in the LibreOffice API has five major architectural areas, cf. Illustration 1 below. The five areas are:
- text
- service manager (document internal)
- draw page
- text content suppliers
- objects for styling and numbering
The core of the text document model is the text. It consists of character strings organized in paragraphs and other text contents. The usage of text will be discussed in Working with Text Documents.
The service manager of the document model creates all text contents for the model, except for the paragraphs. Note that the document service manager is different from the main service manager that is used when connecting to the office. Each document model has its own service manager, so that the services can be adapted to the document when required. Examples for text contents created by the text document service manager are text tables, text fields, drawing shapes, text frames or graphic objects. The service manager is asked for a text content, then you insert it into the text.
Afterwards, the majority of these text contents in a text can be retrieved from the model using text content suppliers. The exception are drawing shapes. They can be found on the DrawPage
, which is discussed below.
Above the text lies the DrawPage
. It is used for drawing contents. Imagine it as a transparent layer with contents that can affect the text under the layer, for instance by forcing it to wrap around contents on the DrawPage
. However, text can also wrap through DrawPage
contents, so the similarity is limited.
Finally, there are services that allow for document wide styling and structuring of the text. Among them are style family suppliers for paragraphs, characters, pages and numbering patterns, and suppliers for line and outline numbering.
Besides these five architectural areas, there are a number of aspects covering the document character of our model: It is printable, storable, modifiable, it can be refreshed, its contents are able to be searched and replaced and it supplies general information about itself. These aspects are shown at the lower right of the illustration.
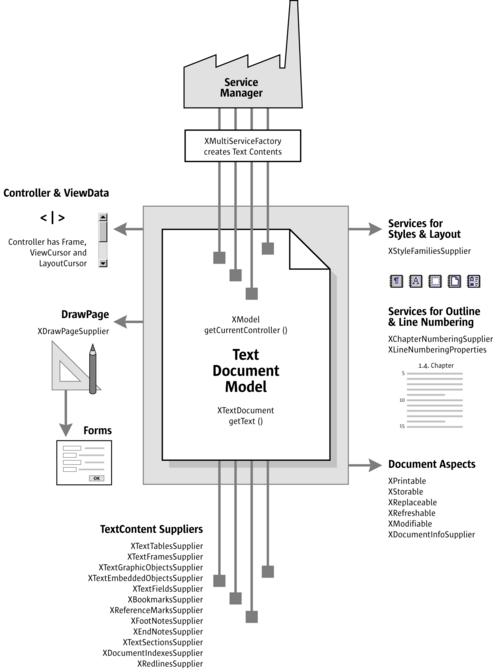
Finally, the controller provides access to the graphical user interface for the model and has knowledge about the current view status in the user interface, cf. the upper left of the diagram above.
The usage of text is discussed in the section Working with Text Documents.
Example: Fields in a Template
All following code samples are contained in TextDocuments.java. This file is located in the Samples folder that comes with the resources for the developer's manual.
The examples use the environment from chapter First Steps, for instance, connecting using the getRemoteServiceManager()
method.
We want to use a template file containing text fields and bookmarks and insert text into the fields and at the cursor position. The suitable template file TextTemplateWithUserFields.odt lies in the Samples folder, as well. Edit the path to this file below before running the sample.
The first step is to load the file as a template, so that LibreOffice creates a new, untitled document. As in the chapter First Steps, we have to connect, get the Desktop object, query its XComponentLoader
interface and call loadComponentFromUrl()
. This time we tell LibreOffice how it should load the file. The key for loading parameters is the sequence of PropertyValue
structs passed to loadComponentFromUrl()
. The appropriate PropertyValue
name is AsTemplate
and we have to set AsTemplate
to true.
/** Load a document as template */
protected XComponent newDocComponentFromTemplate(String loadUrl) throws java.lang.Exception {
// get the remote service manager
mxRemoteServiceManager = this.getRemoteServiceManager(unoUrl);
// retrieve the Desktop object, we need its XComponentLoader
Object desktop = mxRemoteServiceManager.createInstanceWithContext(
"com.sun.star.frame.Desktop", mxRemoteContext);
XComponentLoader xComponentLoader = UnoRuntime.queryInterface(
XComponentLoader.class, desktop);
// define load properties according to com.sun.star.document.MediaDescriptor
// the boolean property AsTemplate tells the office to create a new document
// from the given file
PropertyValue[] loadProps = new PropertyValue[1];
loadProps[0] = new PropertyValue();
loadProps[0].Name = "AsTemplate";
loadProps[0].Value = Boolean.TRUE;
// load
return xComponentLoader.loadComponentFromURL(loadUrl, "_blank", 0, loadProps);
}
Now that we are able to load a text document as a template, we will open an existing template file that contains five text fields and a bookmark. We want to demonstrate how to insert text at predefined positions in a document.
Text fields and bookmarks are supplied by the appropriate XTextFieldsSupplier
and XBookmarksSupplier
interfaces. Their fully qualified names are com.sun.star.text.XTextFieldsSupplier and com.sun.star.text.XBookmarksSupplier.
The XTextFieldsSupplier
provides collections of text fields in our text. We use document variable fields for our purpose, which are com.sun.star.text.textfield.User services. All User fields have a field master that holds the actual content of the variable. Therefore, the TextFields
collection, as well as the FieldMasters
are required for our example. We get the field masters for the five fields by name and set their Content
property. Finally, we refresh the text fields so that they reflect the changes made to the field masters.
The XBookmarksSupplier
returns all bookmarks in our document. The collection of bookmarks is a com.sun.star.container.XNameAccess, so that the bookmarks are retrieved by name. Every object in a text supports the interface XTextContent
that has a method getAnchor()
. The anchor is the text range an object takes up, so getAnchor()
retrieves is a XTextRange
. From the chapter First Steps, a com.sun.star.text.XTextRange allows setting the string of a text range. Our bookmark is a text content and therefore must support XTextContent
. Inserting text at a bookmark position is straightforward: get the anchor of the bookmark and set its string.
/** Sample for use of templates
This sample uses the file TextTemplateWithUserFields.odt from the Samples folder.
The file contains a number of User text fields (Variables - User) and a bookmark
which we use to fill in various values
*/
protected void templateExample() throws java.lang.Exception {
// create a small hashtable that simulates a rowset
HashMap<String,String> recipient = new HashMap<String,String>();
recipient.put("Company", "Manatee Books");
recipient.put("Contact", "Rod Martin");
recipient.put("ZIP", "34567");
recipient.put("City", "Fort Lauderdale");
recipient.put("State", "Florida");
// load template with User fields and bookmark
java.io.File sourceFile = new java.io.File("TextTemplateWithUserFields.odt");
StringBuffer sTemplateFileUrl = new StringBuffer("file:///");
sTemplateFileUrl.append(sourceFile.getCanonicalPath().replace('\\', '/'));
XComponent xTemplateComponent =
newDocComponentFromTemplate( sTemplateFileUrl.toString() );
// get XTextFieldsSupplier, XBookmarksSupplier interfaces
XTextFieldsSupplier xTextFieldsSupplier = UnoRuntime.queryInterface(XTextFieldsSupplier.class,
xTemplateComponent);
XBookmarksSupplier xBookmarksSupplier = UnoRuntime.queryInterface(XBookmarksSupplier.class, xTemplateComponent);
// access the TextFields and the TextFieldMasters collections
XNameAccess xNamedFieldMasters = xTextFieldsSupplier.getTextFieldMasters();
XEnumerationAccess xEnumeratedFields = xTextFieldsSupplier.getTextFields();
// iterate over hashtable and insert values into field masters
for(Iterator<String> iter = recipient.keySet().iterator(); iter.hasNext(); ) {
// get column name
String key = iter.next();
// access corresponding field master
Object fieldMaster = xNamedFieldMasters.getByName(
"com.sun.star.text.fieldmaster.User." + key);
// query the XPropertySet interface, we need to set the Content property
XPropertySet xPropertySet = UnoRuntime.queryInterface(
XPropertySet.class, fieldMaster);
// insert the column value into field master
xPropertySet.setPropertyValue("Content", recipient.get(key));
}
// afterwards we must refresh the textfields collection
XRefreshable xRefreshable = UnoRuntime.queryInterface(
XRefreshable.class, xEnumeratedFields);
xRefreshable.refresh();
// accessing the Bookmarks collection of the document
XNameAccess xNamedBookmarks = xBookmarksSupplier.getBookmarks();
// find the bookmark named "Subscription"
Object bookmark = xNamedBookmarks.getByName("Subscription");
// we need its XTextRange which is available from getAnchor(),
// so query for XTextContent
XTextContent xBookmarkContent = UnoRuntime.queryInterface(
XTextContent.class, bookmark);
// get the anchor of the bookmark (its XTextRange)
XTextRange xBookmarkRange = xBookmarkContent.getAnchor();
// set string at the bookmark position
xBookmarkRange.setString("subscription for the Manatee Journal");
}
Example: Visible Cursor Position
As discussed earlier, the LibreOffice API distinguishes between the model and controller. This difference is mirrored in two different kinds of cursors in the API: model cursors and visible cursors. The visible cursor is also called view cursor.
The second example assumes that the user has selected a text range in a paragraph and expects something to happen at that cursor position. Setting character and paragraph styles, and retrieving the current page number at the view cursor position is demonstrated in the example. The view cursor will be transformed into a model cursor.
We want to work with the current document, therefore we cannot use loadComponentFromURL()
. Rather, we ask the com.sun.star.frame.Desktop service for the current component. Once we have the current component - which is our document model - we go from the model to the controller and get the view cursor.
The view cursor has properties for the current character and paragraph style. The example uses built-in styles and sets the property CharStyleName
to "Quotation
" and ParaStyleName
to "Quotations
". Furthermore, the view cursor knows about the automatic page breaks. Because we are interested in the current page number, we get it from the view cursor and print it out.
The model cursor is much more powerful than the view cursor when it comes to possible movements and editing capabilities. We create a model cursor from the view cursor. Two steps are necessary: We ask the view cursor for its Text service, then we have the Text service create a model cursor based on the current cursor position. The model cursor knows where the paragraph ends, so we go there and insert a string.
/** Sample for document changes, starting at the current view cursor position
The sample changes the paragraph style and the character style at the current
view cursor selection
Open the sample file ViewCursorExampleFile, select some text and run the example
The current paragraph will be set to Quotations paragraph style
The selected text will be set to Quotation character style
*/
private void viewCursorExample() throws java.lang.Exception {
// get the remote service manager
mxRemoteServiceManager = this.getRemoteServiceManager(unoUrl);
// get the Desktop service
Object desktop = mxRemoteServiceManager.createInstanceWithContext(
"com.sun.star.frame.Desktop", mxRemoteContext);
// query its XDesktop interface, we need the current component
XDesktop xDesktop = UnoRuntime.queryInterface(
XDesktop.class, desktop);
// retrieve the current component and access the controller
XComponent xCurrentComponent = xDesktop.getCurrentComponent();
// get the XModel interface from the component
XModel xModel = UnoRuntime.queryInterface(XModel.class, xCurrentComponent);
// the model knows its controller
XController xController = xModel.getCurrentController();
// the controller gives us the TextViewCursor
// query the viewcursor supplier interface
XTextViewCursorSupplier xViewCursorSupplier =
UnoRuntime.queryInterface(
XTextViewCursorSupplier.class, xController);
// get the cursor
XTextViewCursor xViewCursor = xViewCursorSupplier.getViewCursor();
// query its XPropertySet interface, we want to set character and paragraph properties
XPropertySet xCursorPropertySet = UnoRuntime.queryInterface(
XPropertySet.class, xViewCursor);
// set the appropriate properties for character and paragraph style
xCursorPropertySet.setPropertyValue("CharStyleName", "Quotation");
xCursorPropertySet.setPropertyValue("ParaStyleName", "Quotations");
// print the current page number - we need the XPageCursor interface for this
XPageCursor xPageCursor = UnoRuntime.queryInterface(
XPageCursor.class, xViewCursor);
System.out.println("The current page number is " + xPageCursor.getPage());
// the model cursor is much more powerful, so
// we create a model cursor at the current view cursor position with the following steps:
// we get the Text service from the TextViewCursor, the cursor is an XTextRange and has
// therefore a method getText()
XText xDocumentText = xViewCursor.getText();
// the text creates a model cursor from the viewcursor
XTextCursor xModelCursor = xDocumentText.createTextCursorByRange(xViewCursor.getStart());
// now we could query XWordCursor, XSentenceCursor and XParagraphCursor
// or XDocumentInsertable, XSortable or XContentEnumerationAccess
// and work with the properties of com.sun.star.text.TextCursor
// in this case we just go to the end of the paragraph and add some text.
XParagraphCursor xParagraphCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, xModelCursor);
// goto the end of the paragraph
xParagraphCursor.gotoEndOfParagraph(false);
xParagraphCursor.setString(" ***** Fin de semana! ******");
}
Handling Text Document Files
Creating and Loading Text Documents
If a document in LibreOffice is required, begin by getting a com.sun.star.frame.Desktop service from the service manager. The desktop handles all document components in LibreOffice, among other things. It is discussed thoroughly in the chapter Office Development. Office documents are often called components, because they support the com.sun.star.lang.XComponent interface. An XComponent
is a UNO object that can be disposed explicitly and broadcast an event to other UNO objects when this happens.
The Desktop can load new and existing components from a URL. For this purpose it has a com.sun.star.frame.XComponentLoader interface that has one single method to load and instantiate components from a URL into a frame:
com.sun.star.lang::XComponent loadComponentFromURL([in] string aURL,
[in] string aTargetFrameName,
[in] long nSearchFlags,
[in] sequence< com::sun::star::beans::PropertyValue > aArgs );
The interesting parameters in our context are the URL that describes which resource should be loaded and the sequence of load arguments. For the target frame pass "_blank
" and set the search flags to 0. In most cases you will not want to reuse an existing frame.
The URL can be a file:
URL, a http:
URL, an ftp:
URL or a private:
URL. Look up the correct URL format in the load URL box in the function bar of LibreOffice. For new Writer documents, a special URL scheme has to be used. The scheme is "private:", followed by "factory" as hostname. The resource is "swriter" for LibreOffice Writer documents. For a new Writer document, use "private:factory/swriter
".
The load arguments are described in com.sun.star.document.MediaDescriptor. The arguments AsTemplate
and Hidden
have properties that are boolean values. If AsTemplate
is true, the loader creates a new untitled document from the given URL. If it is false, template files are loaded for editing. If Hidden
is true, the document is loaded in the background. This is useful when generating a document in the background without letting the user observe; for example, it can be used to generate a document and print it without previewing. Office development describes other available options.
The section Example: Fields in a Template discusses a complete example about how loading works. The following snippet loads a document in hidden mode:
// (the method getRemoteServiceManager is described in the chapter First Steps)
mxRemoteServiceManager = this.getRemoteServiceManager(unoUrl);
// retrieve the Desktop object, we need its XComponentLoader
Object desktop = mxRemoteServiceManager.createInstanceWithContext(
"com.sun.star.frame.Desktop", mxRemoteContext);
// query the XComponentLoader interface from the Desktop service
XComponentLoader xComponentLoader = UnoRuntime.queryInterface(
XComponentLoader.class, desktop);
// define load properties according to com.sun.star.document.MediaDescriptor
/* or simply create an empty array of com.sun.star.beans.PropertyValue structs:
PropertyValue[] loadProps = new PropertyValue[0]
*/
// the boolean property Hidden tells the office to open a file in hidden mode
PropertyValue[] loadProps = new PropertyValue[1];
loadProps[0] = new PropertyValue();
loadProps[0].Name = "Hidden";
loadProps[0].Value = Boolean.TRUE;
// load
return xComponentLoader.loadComponentFromURL(loadUrl, "_blank", 0, loadProps);
Saving Text Documents
Storing
Documents are storable through their interface com.sun.star.frame.XStorable. This interface is discussed in detail in Office Development. An XStorable
implements these operations:
boolean hasLocation()
string getLocation()
boolean isReadonly()
void store()
void storeAsURL( [in] string aURL, sequence< com::sun::star::beans::PropertyValue > aArgs)
void storeToURL( [in] string aURL, sequence< com::sun::star::beans::PropertyValue > aArgs)
The method names are evident. The method storeAsUrl()
is the exact representation of File - Save As, that is, it changes the current document location. In contrast, storeToUrl()
stores a copy to a new location, but leaves the current document URL untouched.
Exporting
For exporting purposes, a filter name can be passed to storeAsURL()
and storeToURL()
that triggers an export to other file formats. The property needed for this purpose is the string argument FilterName
that takes filter names defined in the configuration file:
<OfficePath>\share\config\registry\instance\org\openoffice\Office\TypeDetection.xml
In TypeDetection.xml, look for <Filter/>
elements, their cfg:name
attribute contains the needed strings for FilterName
. The proper filter name for StarWriter 5.x is "StarWriter 5.0", and the export format "MS Word 97" is also popular. This is the element in TypeDetection.xml that describes the MS Word 97 filter:
<Filter cfg:name="MS Word 97">
<Installed cfg:type="boolean">true</Installed>
<UIName cfg:type="string" cfg:localized="true">
<cfg:value xml:lang="en-US">Microsoft Word 97/2000/XP</cfg:value>
</UIName>
<Data cfg:type="string">3,writer_MS_Word_97,com.sun.star.text.TextDocument,,67,CWW8,0,,</Data>
</Filter>
The following method stores a document using this filter:
/** Store a document, using the MS Word 97/2000/XP Filter */
protected void storeDocComponent(XComponent xDoc, String storeUrl) throws java.lang.Exception {
XStorable xStorable = UnoRuntime.queryInterface(XStorable.class, xDoc);
PropertyValue[] storeProps = new PropertyValue[1];
storeProps[0] = new PropertyValue();
storeProps[0].Name = "FilterName";
storeProps[0].Value = "MS Word 97";
xStorable.storeAsURL(storeUrl, storeProps);
}
If an empty array of PropertyValue
structs is passed, the native .odt format of LibreOffice is used.
Printing Text Documents
Printer and Print Job Settings
Printing is a common office functionality. The chapter Office Development provides in-depth information about it. The writer document implements the com.sun.star.view.XPrintable interface for printing. It consists of three methods:
sequence< com::sun::star::beans::PropertyValue > getPrinter ()
void setPrinter ( [in] sequence< com::sun::star::beans::PropertyValue > aPrinter)
void print ( [in] sequence< com::sun::star::beans::PropertyValue > xOptions)
The following code is used with a given document xDoc to print to the standard printer without any settings:
// query the XPrintable interface from your document
XPrintable xPrintable = UnoRuntime.queryInterface(XPrintable.class, xDoc);
// create an empty printOptions array
PropertyValue[] printOpts = new PropertyValue[0];
// kick off printing
xPrintable.print(printOpts);
There are two groups of properties involved in general printing. The first one is used with setPrinter()
and getPrinter()
that controls the printer, and the second one is passed to print()
and controls the print job.
com.sun.star.view.PrinterDescriptor comprises the properties for the printer:
Properties of com.sun.star.view.PrinterDescriptor | |
---|---|
Name | string - Specifies the name of the printer queue to be used.
|
PaperOrientation | com.sun.star.view.PaperOrientation. Specifies the orientation of the paper. |
PaperFormat | com.sun.star.view.PaperFormat. Specifies a predefined paper size or if the paper size is a user-defined size. |
PaperSize | com.sun.star.awt.Size. Specifies the size of the paper in 1/100 mm. |
IsBusy | boolean - Indicates if the printer is busy.
|
CanSetPaperOrientation | boolean - Indicates if the printer allows changes to PaperOrientation .
|
CanSetPaperFormat | boolean - Indicates if the printer allows changes to PaperFormat .
|
CanSetPaperSize | boolean - Indicates if the printer allows changes to PaperSize .
|
com.sun.star.view.PrintOptions contains the following possibilities for a print job:
Properties of com.sun.star.view.PrintOptions | |
---|---|
CopyCount | short - Specifies the number of copies to print.
|
FileName | string - Specifies the name of a file to print to, if set.
|
Collate | boolean - Advises the printer to collate the pages of the copies. If true, a whole document is printed prior to the next copy, otherwise the page copies are completed together.
|
Pages | string - Specifies the pages to print in the same format as in the print dialog of the GUI (e.g. "1, 3, 4-7, 9-")
|
Wait | boolean - Advises that the print job should be performed synchronously, i.e. wait until printing is complete before returning from printing. Otherwise return is immediate and following actions (e.g. closing the corresponding model) may fail until printing is complete. Default is false.
|
The following method uses PrinterDescriptor
and PrintOptions
to print to a special printer, and preselect the pages to print.
protected void printDocComponent(XComponent xDoc) throws java.lang.Exception {
XPrintable xPrintable = UnoRuntime.queryInterface(XPrintable.class, xDoc);
PropertyValue[] printerDesc = new PropertyValue[1];
printerDesc[0] = new PropertyValue();
printerDesc[0].Name = "Name";
printerDesc[0].Value = "5D PDF Creator";
xPrintable.setPrinter(printerDesc);
PropertyValue[] printOpts = new PropertyValue[1];
printOpts[0] = new PropertyValue();
printOpts[0].Name = "Pages";
printOpts[0].Value = "3-5,7";
xPrintable.print(printOpts);
}
Printing Multiple Pages on one Page
The interface com.sun.star.text.XPagePrintable is used to print more than one document page to a single printed page.
sequence< com::sun::star::beans::PropertyValue > getPagePrintSettings()
void setPagePrintSettings( [in] sequence< com::sun::star::beans::PropertyValue > aSettings)
void printPages( [in] sequence< com::sun::star::beans::PropertyValue > xOptions)
The first two methods getPagePrintSettings()
and setPagePrintSettings()
control the page printing. They use a sequence of com.sun.star.beans.PropertyValues whose possible values are defined in com.sun.star.text.PagePrintSettings:
Properties of com.sun.star.text.PagePrintSettings | |
---|---|
PageRows | short - Number of rows in which document pages should appear on the output page.
|
PageColumns | short - Number of columns in which document pages should appear on the output page.
|
LeftMargin | long - Left margin on the output page.
|
RightMargin | long - Right margin on the output page.
|
TopMargin | long - Top margin on the output page.
|
BottomMargin | long - Bottom margin on the output page.
|
HoriMargin | long - Margin between the columns on the output page.
|
VertMargin | long - Margin between the rows on the output page.
|
IsLandscape | boolean - Determines if the output page is in landscape format.
|
The method printPages()
prints the document according to the previous settings. The argument for the printPages()
method may contain the PrintOptions
as described in the section above (containing the properties CopyCount
, FileName
, Collate
and Pages
).
Working with Text Documents
Word Processing
The text model in the illustration below shows that working with text starts with the method getText()
at the XTextDocument
interface of the document model. It returns a com.sun.star.text.Text service that handles text in LibreOffice.
The Text service has two mandatory interfaces and no properties:

The XText
is used to edit a text, and XEnumerationAccess
is used to iterate over text. The following sections discuss these aspects of the Text service.
Editing Text
As previously discussed in the introductory chapter First Steps, the interface com.sun.star.text.XText incorporates three interfaces: XText
, XSimpleText
and XTextRange
. When working with an XText
, you work with the string it contains, or you insert and remove contents other than strings, such as tables, text fields, and graphics.
Strings
The XText
is handled as a whole. There are two possibilities if the text is handled as one string. The complete string can be set at once, or strings can be added at the beginning or end of the existing text. These are the appropriate methods used for that purpose:
void setString( [in] string text)
String getString()
Consider the following example:
/** Setting the whole text of a document as one string */
protected void BodyTextExample() {
// Body Text and TextDocument example
try {
// demonstrate simple text insertion
mxDocText.setString("This is the new body text of the document."
+ "\n\nThis is on the second line.\n\n");
} catch (Exception e) {
e.printStackTrace (System.out);
}
}
Beginning and end of a text can be determined calling getStart()
and getEnd()
:
com::sun::star::text::XTextRange getStart()
com::sun::star::text::XTextRange getEnd()
The following example adds text using the start and end range of a text:
/** Adding a string at the end or the beginning of text */
protected void TextRangeExample() {
try {
// Get a text range referring to the beginning of the text document
XTextRange xStart = mxDocText.getStart();
// use setString to insert text at the beginning
xStart.setString ("This is text inserted at the beginning.\n\n");
// Get a text range referring to the end of the text document
XTextRange xEnd = mxDocText.getEnd();
// use setString to insert text at the end
xEnd.setString ("This is text inserted at the end.\n\n");
} catch (Exception e) {
e.printStackTrace(System.err);
}
}
The above code is not very flexible. To gain flexibility, create a text cursor that is a movable text range. Note that such a text cursor is not visible in the user interface. The XText
creates a cursor that works on the model immediately. The following methods can be used to get as many cursors as required:
com::sun::star::text::XTextCursor createTextCursor() com::sun::star::text::XTextCursor createTextCursorByRange ( com::sun::star::text::XTextRange aTextPosition)
The text cursor travels through the text as a "collapsed" text range with identical start and end as a point in text, or it can expand while it moves to contain a target string. This is controlled with the methods of the XTextCursor
interface:
// moving the cursor // if bExpand is true, the cursor expands while it travels boolean goLeft( [in] short nCount, [in] boolean bExpand) boolean goRight( [in] short nCount, [in] boolean bExpand) void gotoStart( [in] boolean bExpand) void gotoEnd( [in] boolean bExpand) void gotoRange( [in] com::sun::star::text::XTextRange xRange, [in] boolean bExpand)
// controlling the collapsed status of the cursor void collapseToStart() void collapseToEnd() boolean isCollapsed()
In writer, a text cursor has three interfaces that inherit from XTextCursor
: com.sun.star.text.XWordCursor, com.sun.star.text.XSentenceCursor and com.sun.star.text.XParagraphCursor. These interfaces introduce the following additional movements and status checks:
boolean gotoNextWord( [in] boolean bExpand) boolean gotoPreviousWord( [in] boolean bExpand) boolean gotoEndOfWord( [in] boolean bExpand) boolean gotoStartOfWord( [in] boolean bExpand) boolean isStartOfWord() boolean isEndOfWord()
boolean gotoNextSentence( [in] boolean Expand) boolean gotoPreviousSentence( [in] boolean Expand) boolean gotoStartOfSentence( [in] boolean Expand) boolean gotoEndOfSentence( [in] boolean Expand) boolean isStartOfSentence() boolean isEndOfSentence()
boolean gotoStartOfParagraph( [in] boolean bExpand) boolean gotoEndOfParagraph( [in] boolean bExpand) boolean gotoNextParagraph( [in] boolean bExpand) boolean gotoPreviousParagraph( [in] boolean bExpand) boolean isStartOfParagraph() boolean isEndOfParagraph()
Since XTextCursor
inherits from XTextRange
, a cursor is an XTextRange
and incorporates the methods of an XTextRange
:
com::sun::star::text::XText getText() com::sun::star::text::XTextRange getStart() com::sun::star::text::XTextRange getEnd() string getString() void setString( [in] string aString)
The cursor can be told where it is required and the string content can be set later. This does have a drawback. After setting the string, the inserted string is always selected. That means further text can not be added without moving the cursor again. Therefore the most flexible method to insert strings by means of a cursor is the method insertString()
in XText
. It takes an XTextRange
as the target range that is replaced during insertion, a string to insert, and a boolean parameter that determines if the inserted text should be absorbed by the cursor after it has been inserted. The XTextRange
could be any XTextRange
. The XTextCursor
is an XTextRange
, so it is used here:
void insertString( [in] com::sun::star::text::XTextRange xRange, [in] string aString, [in] boolean bAbsorb)
To insert text sequentially the bAbsorb
parameter must be set to false, so that the XTextRange
collapses at the end of the inserted string after insertion. If bAbsorb
is true, the text range selects the new inserted string. The string that was selected by the text range prior to insertion is deleted.
Consider the use of insertString()
below:
/** moving a text cursor, selecting text and overwriting it */
protected void TextCursorExample() {
try {
// First, get the XSentenceCursor interface of our text cursor
XSentenceCursor xSentenceCursor = UnoRuntime.queryInterface(
XSentenceCursor.class, mxDocCursor);
// Goto the next cursor, without selecting it
xSentenceCursor.gotoNextSentence(false);
// Get the XWordCursor interface of our text cursor
XWordCursor xWordCursor = UnoRuntime.queryInterface(
XWordCursor.class, mxDocCursor);
// Skip the first four words of this sentence and select the fifth
xWordCursor.gotoNextWord(false);
xWordCursor.gotoNextWord(false);
xWordCursor.gotoNextWord(false);
xWordCursor.gotoNextWord(false);
xWordCursor.gotoNextWord(true);
// Use the XSimpleText interface to insert a word at the current cursor
// location, over-writing
// the current selection (the fifth word selected above)
mxDocText.insertString(xWordCursor, "old ", true);
// Access the property set of the cursor, and set the currently selected text
// (which is the string we just inserted) to be bold
XPropertySet xCursorProps = UnoRuntime.queryInterface(
XPropertySet.class, mxDocCursor);
xCursorProps.setPropertyValue("CharWeight", new Float(com.sun.star.awt.FontWeight.BOLD));
// replace the '.' at the end of the sentence with a new string
xSentenceCursor.gotoEndOfSentence(false);
xWordCursor.gotoPreviousWord(true);
mxDocText.insertString(xWordCursor,
", which has been changed with text cursors!", true);
} catch (Exception e) {
e.printStackTrace(System.err);
}
}
Text Contents Other Than Strings
Up to this point, we have discussed paragraphs made up of character strings. Text can also contain other objects besides character strings in paragraphs. They all support the interface com.sun.star.text.XTextContent. In fact, everything in texts must support XTextContent
.
A text content is an object that is attached to a com.sun.star.text.XTextRange. The text range it is attached to is called the anchor of the text content.
All text contents mentioned below, starting with tables, support the service com.sun.star.text.TextContent. It includes the interface com.sun.star.text.XTextContent that inherits from the interface com.sun.star.lang.XComponent. The TextContent
services may have the following properties:
Properties of com.sun.star.text.TextContent | |
---|---|
AnchorType | Describes the base the object is positioned to, according to com.sun.star.text.TextContentAnchorType. |
AnchorTypes | A sequence of com.sun.star.text.TextContentAnchorType that contains all allowed anchor types for the object. |
TextWrap | Determines the way the surrounding text flows around the object, according to com.sun.star.text.WrapTextMode. |
The method dispose()
of the XComponent
interface deletes the object from the document. Since a text content is an XComponent
, com.sun.star.lang.XEventListener can be added or removed with the methods addEventListener()
and removeEventListener()
. These methods are called back when the object is disposed. Other events are not supported.
The method getAnchor()
at the XTextContent
interface returns a text range which reflects the text position where the object is located. This method may return a void object, for example, for text frames that are bound to a page. The method getAnchor()
is used in situations where an XTextRange
is required. For instance, placeholder fields (com.sun.star.text.textfield.JumpEdit) can be filled out using their getAnchor()
method. Also, you can get a bookmark, retrieve its XTextRange
from getAnchor()
and use it to insert a string at the bookmark position.
The method attach()
is an intended method to attach text contents to the document, but it is currently not implemented.
All text contents - including paragraphs - can be created by the service manager of the document. They are created using the factory methods createInstance()
or createInstanceWithArguments()
at the com.sun.star.lang.XMultiServiceFactory interface of the document.
All text contents - except for paragraphs - can be inserted into text using the com.sun.star.text.XText method insertTextContent()
. They can be removed by calling removeTextContent()
. Starting with the section Tables, there are code samples showing the usage of the document service manager with insertTextContent()
.
void insertTextContent( [in] com::sun::star::text::XTextRange xRange, [in] com::sun::star::text::XTextContent xContent, boolean bAbsorb); void removeTextContent( [in] com::sun::star::text::XTextContent xContent)
Paragraphs cannot be inserted by insertTextContent()
. Only the interface XRelativeTextContentInsert
can insert paragraphs. A paragraph created by the service manager can be used for creating a new paragraph before or after a table, or a text section positioned at the beginning or the end of page where no cursor can insert new paragraphs. Cf. the section Inserting a Paragraph where no Cursor can go below.
Control Characters
We have used Java escape sequences for paragraph breaks, but this may not be feasible in every language. Moreover, LibreOffice supports a number of control characters that can be used. There are two possibilities: use the method
void insertControlCharacter( [in] com::sun::star::text::XTextRange xRange, [in] short nControlCharacter, [in] boolean bAbsorb)
to insert single control characters as defined in the constants group com.sun.star.text.ControlCharacter, or use the corresponding unicode character from the following list as escape sequence in a string if your language supports it. In Java, Unicode characters in strings can be incorporated using the \uHHHH
escape sequence, where H represents a hexadecimal digit
PARAGRAPH_BREAK
|
Insert a paragraph break (UNICODE 0x000D ).
|
LINE_BREAK
|
Inserts a line break inside the paragraph (UNICODE 0x000A ).
|
HARD_HYPHEN
|
A character that appears like a dash, but prevents hyphenation at its position (UNICODE 0x2011 ).
|
SOFT_HYPHEN
|
Marks a preferred position for hyphenation (UNICODE 0x00AD ).
|
HARD_SPACE
|
A character that appears like a space, but prevents hyphenation at this point (UNICODE 0x00A0 ).
|
APPEND_PARAGRAPH
|
A new paragraph is appended (no UNICODE for this function). |
The section Formatting describes how page breaks are created by setting certain paragraph properties.
Iterating over Text
The second interface of com.sun.star.text.Text is XEnumerationAccess
. A Text service enumerates all paragraphs in a text and returns objects which support com.sun.star.text.Paragraph. This includes tables, because writer sees tables as specialized paragraphs that support the com.sun.star.text.TextTable service.
Paragraphs also have an com.sun.star.container.XEnumerationAccess of their own. They can enumerate every single text portion that they contain. A text portion is a text range containing a uniform piece of information that appears within the text flow. An ordinary paragraph, formatted in a uniform manner and containing nothing but a string, enumerates just a single text portion. In a paragraph that has specially formatted words or other contents, the text portion enumeration returns one com.sun.star.text.TextPortion service for each differently formatted string, and for every other text content. Text portions include the service com.sun.star.text.TextRange and have the properties listed below:
Properties of com.sun.star.text.TextPortion | |
---|---|
TextPortionType | string - Contains the type of the text portion (see below).
|
ControlCharacter | short - Returns the control character if the text portion contains a control character as defined in com.sun.star.text.ControlCharacter.
|
Bookmark | com.sun.star.text.XTextContent. Contains the bookmark if the portion has TextPortionType="Bookmark". |
DocumentIndexMark | com.sun.star.text.XTextContent. Contains the document index mark if the portion has TextPortionType="DocumentIndexMark". |
ReferenceMark | com.sun.star.text.XTextContent. Contains the reference mark if the portion has TextPortionType="ReferenceMark". |
Footnote | com.sun.star.text.XFootnote. Contains the footnote if the portion has TextPortionType="Footnote". |
TextField | com.sun.star.text.XTextContent. Contains the text field if the portion has TextPortionType="TextField". |
InContentMetadata | com.sun.star.text.XTextContent. Contains the text range if the portion has TextPortionType="InContentMetadata". |
IsCollapsed | boolean - Contains whether the portion is a point only.
|
IsStart | boolean - Contains whether the portion is a start portion if two portions are needed to include an object, for example, DocumentIndexMark .
|
Possible Values for TextPortionType
are:
TextPortionType (String) | Description |
---|---|
"Text" | a portion with mere string content |
"TextField" | A com.sun.star.text.TextField content. |
"TextContent" | A text content supplied through the interface XContentEnumerationAccess .
|
"Footnote" | A footnote or an endnote. |
"ControlCharacter" | A control character. |
"ReferenceMark" | A reference mark. |
"DocumentIndexMark" | A document index mark. |
"Bookmark" | A bookmark. |
"Redline" | A redline portion which is a result of the change tracking feature. |
"Ruby" | A ruby attribute which is used in Asian text. |
"Frame" | A frame supplied through the interface XContentEnumerationAccess .
|
"SoftPageBreak" | A soft page break. |
"InContentMetadata" | A text range with attached metadata. |
The text portion enumeration of a paragraph does not supply contents which do belong to the paragraph, but do not fuse together with the text flow. These could be text frames, graphic objects, embedded objects or drawing shapes anchored at the paragraph, characters or as character. The TextPortionType
"TextContent
" indicate if there is a content anchored at a character or as a character. If you have a TextContent
portion type, you know that there are shape objects anchored at a character or as a character.
This last group of data contained in a text, Paragraphs
and TextPortions
in writer support the interface com.sun.star.container.XContentEnumerationAccess. This interface tells which text contents other than the text flow contents exist, and supplies them as an com.sun.star.container.XEnumeration:
sequence< string > getAvailableServiceNames() com::sun::star::container::XEnumeration createContentEnumeration( [in] string aServiceName)
The XContentEnumerationAccess
of the paragraph lists the shape objects anchored at the paragraph while the XContentEnumerationAccess
of a text portion lists the shape objects anchored at a character or as a character.
The enumeration access to text through paragraphs and text portions is used if every single paragraph in a text needs to be touched. The application area for this enumeration are export filters, that uses this enumeration to go over the whole document, writing out the paragraphs to the target file. The following code snippet centers all paragraphs in a text.
/** This method demonstrates how to iterate over paragraphs */
protected void ParagraphExample () {
try {
// The service 'com.sun.star.text.Text' supports the XEnumerationAccess interface to
// provide an enumeration
// of the paragraphs contained by the text the service refers to.
// Here, we access this interface
XEnumerationAccess xParaAccess = UnoRuntime.queryInterface(
XEnumerationAccess.class, mxDocText);
// Call the XEnumerationAccess's only method to access the actual Enumeration
XEnumeration xParaEnum = xParaAccess.createEnumeration();
// While there are paragraphs, do things to them
while (xParaEnum.hasMoreElements()) {
// Get a reference to the next paragraphs XServiceInfo interface. TextTables
// are also part of this enumeration access, so we ask the element if it is
// a TextTable, if it doesn't support the com.sun.star.text.TextTable
// service, then it is safe to assume that it really is a paragraph
XServiceInfo xInfo = UnoRuntime.queryInterface(
XServiceInfo.class, xParaEnum.nextElement());
if (!xInfo.supportsService("com.sun.star.text.TextTable")) {
// Access the paragraph's property set...the properties in this
// property set are listed
// in: com.sun.star.style.ParagraphProperties
XPropertySet xSet = UnoRuntime.queryInterface(
XPropertySet.class, xInfo);
// Set the justification to be center justified
xSet.setPropertyValue("ParaAdjust", com.sun.star.style.ParagraphAdjust.CENTER);
}
}
} catch (Exception e) {
e.printStackTrace (System.out);
}
}
Inserting a Paragraph where no Cursor can go
The service com.sun.star.text.Text has an optional interface com.sun.star.text.XRelativeTextContentInsert which is available in Text services in Writer. The intention of this interface is to insert paragraphs in positions where no cursor or text portion can be located to use the insertTextContent()
method. These situation occurs when text sections or text tables are at the start or end of the document, or if they follow each other directly.
void insertTextContentBefore( [in] com::sun::star::text::XTextContent xNewContent, [in] com::sun::star::text::XTextContent xSuccessor) void insertTextContentAfter( [in] com::sun::star::text::XTextContent xNewContent, [in] com::sun::star::text::XTextContent xPredecessor)
The only supported text contents are com.sun.star.text.Paragraph as new content, and com.sun.star.text.TextSection and com.sun.star.text.TextTable as successor or predecessor.
Sorting Text
It is possible to sort text or the content of text tables.
Sorting of text is done by the text cursor that supports com.sun.star.util.XSortable. It contains two methods:
sequence< com::sun::star::beans::PropertyValue > createSortDescriptor() void sort( [in] sequence< com::sun::star::beans::PropertyValue > xDescriptor)
The method createSortDescriptor()
returns a sequence of com.sun.star.beans.PropertyValue that provides the elements as described in the service com.sun.star.text.TextSortDescriptor
The method sort()
sorts the text that is selected by the cursor, by the given parameters.
Sorting of tables happens directly at the table service, which supports XSortable
. Sorting is a common feature of LibreOffice and it is described in detail in Office Development.
Inserting Text Files
The text cursor in writer supports the interface com.sun.star.document.XDocumentInsertable which has a single method to insert a file at the current cursor position:
void insertDocumentFromURL( [in] string aURL, [in] sequence< com::sun::star::beans::PropertyValue > aOptions)
Pass an URL and an empty sequence of PropertyValue
structs. However, load properties could be used as described in com.sun.star.document.MediaDescriptor.
Auto Text
The auto text function can be used to organize reusable text passages. They allow storing text, including the formatting and all other contents in a text block collection to apply them later. Three services deal with auto text in LibreOffice:
- com.sun.star.text.AutoTextContainer specifies the entire collection of auto texts
- com.sun.star.text.AutoTextGroup describes a category of auto texts
- com.sun.star.text.AutoTextEntry is a single auto text.
/** Insert an autotext at the current cursor position of given cursor mxDocCursor*/
// Get an XNameAccess interface to all auto text groups from the document factory
XNameAccess xContainer = UnoRuntime.queryInterface(
XNameAccess.class, mxFactory.createInstance("com.sun.star.text.AutoTextContainer"));
// Get the autotext group Standard
xGroup = UnoRuntime.queryInterface(
XAutoTextGroup.class, xContainer.getByName("Standard"));
// get the entry Best Wishes (BW)
XAutoTextEntry xEntry = UnoRuntime.queryInterface (
XAutoTextEntry.class, xGroup.getByName ("BW"));
// insert the modified autotext block at the cursor position
xEntry.applyTo(mxDocCursor);
/** Add a new autotext entry to the AutoTextContainer
*/
// Select the last paragraph in the document
xParaCursor.gotoPreviousParagraph(true);
// Get the XAutoTextContainer interface of the AutoTextContainer service
XAutoTextContainer xAutoTextCont = UnoRuntime.queryInterface(
XAutoTextContainer.class, xContainer );
// If the APIExampleGroup already exists, remove it so we can add a new one
if (xContainer.hasByName("APIExampleGroup"))
xAutoTextCont.removeByName("APIExampleGroup" );
// Create a new auto-text group called APIExampleGroup
XAutoTextGroup xNewGroup = xAutoTextCont.insertNewByName ( "APIExampleGroup" );
// Create and insert a new auto text entry containing the current cursor selection
XAutoTextEntry xNewEntry = xNewGroup.insertNewByName(
"NAE", "New AutoTextEntry", xParaCursor);
// Get the XSimpleText and XText interfaces of the new autotext block
XSimpleText xSimpleText = UnoRuntime.queryInterface(
XSimpleText.class, xNewEntry);
XText xText = UnoRuntime.queryInterface(XText.class, xNewEntry);
// Insert a string at the beginning of the autotext block
xSimpleText.insertString(xText.getStart(),
"This string was inserted using the API!\n\n", false);
The current implementation forces the user to close the AutoTextEntry
instance when they are changed, so that the changes can take effect. However, the new AutoText
is not written to disk until the destructor of the AutoTextEntry
instance inside the writer is called. When this example has finished executing, the file on disk correctly contains the complete text "This string was inserted using the API!\n\nSome text for a new autotext block
", but there is no way in Java to call the destructor. It is not clear when the garbage collector deletes the object and writes the modifications to disk.
Formatting
A multitude of character, paragraph and other properties are available for text in LibreOffice. However, the objects implemented in the writer do not provide properties that support com.sun.star.beans.XPropertyChangeListener or com.sun.star.beans.XVetoableChangeListener yet.
Character and paragraph properties are available in the following services:
Services supporting Character and Paragraph Properties | Remark |
---|---|
com.sun.star.text.TextCursor | If collapsed, the CharacterProperties refer to the position on the right hand side of the cursor.
|
com.sun.star.text.Paragraph | |
com.sun.star.text.TextPortion | |
com.sun.star.text.TextTableCursor | |
com.sun.star.text.Shape | |
com.sun.star.table.CellRange | In text tables. |
com.sun.star.text.TextDocument | The model offers a selected number of character properties which apply to the entire document. These are: CharFontName , CharFontStyleName , CharFontFamily , CharFontCharSet , CharFontPitch and their Asian counterparts CharFontStyleNameAsian , CharFontFamilyAsian , CharFontCharSetAsian , CharFontPitchAsian .
|
The character properties are described in the services com.sun.star.style.CharacterProperties, com.sun.star.style.CharacterPropertiesAsian and com.sun.star.style.CharacterPropertiesComplex.
com.sun.star.style.CharacterProperties describes common character properties for all language zones and character properties in Western text. The following table provides possible values.
Properties of com.sun.star.style.CharacterProperties | |
---|---|
CharFontName | string - This property specifies the name of the font in western text.
|
CharFontStyleName | string - This property contains the name of the font style.
|
CharFontFamily | short - This property contains font family that is specified in com.sun.star.awt.FontFamily. Possible values are: DONTKNOW, DECORATIVE, MODERN, ROMAN, SCRIPT, SWISS, and SYSTEM.
|
CharFontCharSet | short - This property contains the text encoding of the font that is specified in com.sun.star.awt.CharSet. Possible values are: DONTKNOW, ANSI MAC, IBMPC_437, IBMPC_850, IBMPC_860, IBMPC_861, IBMPC_863, IBMPC_865, and SYSTEM SYMBOL.
|
CharFontPitch | short - This property contains the font pitch that is specified in com.sun.star.awt.FontPitch. The word font pitch refers to characters per inch, but the possible values are DONTKNOW, FIXED and VARIABLE. VARIABLE points to the difference between proportional and unproportional fonts.
|
CharColor | long - This property contains the value of the text color in ARGB notation. ARGB has four bytes denoting alpha, red, green and blue. In hex notation, this can be used conveniently: 0xAARRGGBB . The AA (Alpha) can be 00 or left out.
|
CharEscapement | [optional] short - Property which contains the relative value of the raisement or lowerment of characters for superscript or subscript styling. Would typically be a positive value for superscript, and a negative value for subscript.
|
CharHeight | float - This value contains the height of the characters in point.
|
CharUnderline | short - This property contains the value for the character underline that is specified in com.sun.star.awt.FontUnderline. A lot of underline types are available. Some possible values are SINGLE, DOUBLE, and DOTTED.
|
CharWeight | float - This property contains the value of the font weight, cf. [com.sun.star.awt.FontWeight. A lot of weights are possible. The common ones are BOLD and NORMAL.
|
CharPosture | long - This property contains the posture of the font as defined in com.sun.star.awt.FontSlant. The most common values are ITALIC and NONE.
|
CharAutoKerning | [optional] boolean - Property to determine whether the kerning tables from the current font are used.
|
CharBackColor | [optional] long - Property which contains the text background color in ARGB: 0xAARRGGBB .
|
CharBackTransparent | [optional] boolean - Determines if the text background color is set to transparent.
|
CharCaseMap | [optional] short - Property which contains the value of the case-mapping of the text for formatting and displaying. Possible CaseMaps are NONE, UPPERCASE, LOWERCASE, TITLE, and SMALLCAPS as defined in the constants group com.sun.star.style.CaseMap. (optional)
|
CharCrossedOut | [optional] boolean - This property is true if the characters are crossed out.
|
CharFlash | [optional] boolean - If this optional property is true , then the characters are flashing
|
CharStrikeout | [optional] short - Determines the type of the strikethrough of the character as defined in com.sun.star.awt.FontStrikeout. Values are NONE, SINGLE, DOUBLE, DONTKNOW, BOLD, and SLASH X.
|
CharWordMode | [optional] boolean - If this property is true , the underline and strike-through properties are not applied to white spaces.
|
CharKerning | [optional] short - Property which contains the value of the kerning of the characters.
|
CharLocale | struct com.sun.star.lang.Locale. Contains the locale (language and country) of the characters.
|
CharKeepTogether | [optional] boolean - Property which marks a range of characters to prevent it from being broken into two lines.
|
CharNoLineBreak | [optional] boolean - Property which marks a range of characters to ignore a line break in this area.
|
CharShadowed | [optional] boolean - True if the characters are formatted and displayed with a shadow effect. (optional)
|
CharFontType | [optional] short - Property which specifies the fundamental technology of the font as specified in com.sun.star.awt.FontType. Possible values are DONTKNOW, RASTER, DEVICE, and SCALABLE.
|
CharStyleName | [optional] string - Specifies the name of the style of the font.
|
CharContoured | [optional] boolean - True if the characters are formatted and displayed with a contour effect.
|
CharCombineIsOn | [optional] boolean - True if text is formatted in two lines.
|
CharCombinePrefix | [optional] string - Contains the prefix string (usually parenthesis) before text that is formatted in two lines.
|
CharCombineSuffix | [optional] string - Contains the suffix string (usually parenthesis) after text that is formatted in two lines.
|
CharEmphasis | [optional] short - Contains the font emphasis value com.sun.star.text.FontEmphasis.
|
CharRelief | [optional] short - Contains the relief value as FontRelief.
|
RubyText | [optional] string - Contains the text that is set as ruby.
|
RubyAdjust | [optional] short - Determines the adjustment of the ruby text as RubyAdjust.
|
RubyCharStyleName | [optional] string - Contains the name of the character style that is applied to RubyText (optional).
|
RubyIsAbove | [optional] boolean - Determines whether the ruby text is printed above/left or below/right of the text (optional) .
|
CharRotation | [optional] short - Determines the rotation of a character in degree.
|
CharRotationIsFitToLine | [optional] short - Determines whether the text formatting tries to fit rotated text into the surrounded line height.
|
CharScaleWidth | [optional] short - Determines the percentage value of scaling of characters.
|
HyperLinkURL | [optional] string - contains the URL of a hyperlink if the URL is set.
|
HyperLinkTarget | [optional] string - contains the name of the target for a hyperlink if the target is set.
|
HyperLinkName | [optional] string - contains the name of the hyperlink if the name is set.
|
VisitedCharStyleName | [optional] string - Contains the character style name for visited hyperlinks.
|
UnvisitedCharStyleName | [optional] string - Contains the character style name for unvisited hyperlinks.
|
CharEscapementHeight | [optional] byte - This is the relative height of subscript or superscript characters in units of percent. A value of 100 would be the original height of the characters.
|
CharNoHyphenation | [optional] boolean - True if the word can be hyphenated at the character.
|
CharUnderlineColor | Color - Gives the color of the underline for that character. |
CharUnderlineHasColor | boolean - True if the CharunderlineColor is used for an underline
|
CharStyleNames | [optional] sequence<string> - specifies the names of the all styles applied to the font.
|
CharHidden | [optional] boolean - True if the characters are invisible
|
TextUserDefinedAttributes | [optional] XNameContainer - This property stores xml attributes. They will be saved to and restored from automatic styles inside xml files.
|
com.sun.star.style.CharacterPropertiesAsian describes properties used in Asian text. All of these properties have a counterpart in CharacterProperties
. They apply as soon as a text is recognized as Asian by the employed Unicode character subset.
Properties of com.sun.star.style.CharacterPropertiesAsian | |
---|---|
CharHeightAsian | float - This value contains the height of the characters in point.
|
CharWeightAsian | float - This property contains the value of the font weight.
|
CharFontNameAsian | string - This property specifies the name of the font style.
|
CharFontStyleNameAsian | string - This property contains the name of the font style.
|
CharFontFamilyAsian | short - This property contains the font family that is specified in com.sun.star.awt.FontFamily.
|
CharFontCharSetAsian | short - This property contains the text encoding of the font that is specified in com.sun.star.awt.CharSet.
|
CharFontPitchAsian | short - This property contains the font pitch that is specified in com.sun.star.awt.FontPitch.
|
CharPostureAsian | long - This property contains the value of the posture of the font as defined in com.sun.star.awt.FontSlant.
|
CharLocaleAsian | struct com.sun.star.lang.Locale - Contains the value of the locale. |
The complex properties com.sun.star.style.CharacterPropertiesComplex refer to the same character settings as in CharacterPropertiesAsian
, only they have the suffix "Complex" instead of "Asian".
com.sun.star.style.ParagraphProperties comprises paragraph properties.
Properties of com.sun.star.style.ParagraphProperties | |
---|---|
ParaAdjust | long - Determines the adjustment of a paragraph.
|
ParaLineSpacing | [optional] struct com.sun.star.style.LineSpacing - Determines the line spacing of a paragraph.
|
ParaBackColor | [optional] long - Contains the paragraph background color.
|
ParaBackTransparent | [optional] boolean - This value is true if the paragraph background color is set to transparent.
|
ParaBackGraphicURL | [optional] string - Contains the value of a link for the background graphic of a paragraph.
|
ParaBackGraphicFilter | [optional] string - Contains the name of the graphic filter for the background graphic of a paragraph.
|
ParaBackGraphicLocation | [optional] long - Contains the value for the position of a background graphic according to com.sun.star.style.GraphicLocation.
|
ParaLastLineAdjust | short - Determines the adjustment of the last line.
|
ParaExpandSingleWord | [optional] boolean - Determines if single words are stretched.
|
ParaLeftMargin | long - Determines the left margin of the paragraph in 1/100 mm.
|
ParaRightMargin | long - Determines the right margin of the paragraph in 1/100 mm.
|
ParaTopMargin | long - Determines the top margin of the paragraph in 1/100 mm.
|
ParaBottomMargin | long - Determines the bottom margin of the paragraph in 1/100 mm.
|
ParaLineNumberCount | [optional] boolean - Determines if the paragraph is included in the line numbering.
|
ParaLineNumberStartValue | [optional] boolean - Contains the start value for the line numbering.
|
ParaIsHyphenation | [optional] boolean - Prevents the paragraph from getting hyphenated.
|
PageDescName | [optional] string - If this property is set, it creates a page break before the paragraph it belongs to and assigns the value as the name of the new page style sheet to use.
|
PageNumberOffset | [optional] short - If a page break property is set at a paragraph, this property contains the new value for the page number.
|
PageStyleName | [optional] string - Contains the page style name.
|
ParaRegisterModeActive | [optional] boolean - Determines if the register mode is applied to a paragraph.
|
ParaTabStops | [optional] sequence < com.sun.star.style.TabStop > . Specifies the positions and kinds of the tab stops within this paragraph.
|
ParaStyleName | [optional] string - Contains the name of the current paragraph style.
|
DropCapFormat | [optional] struct com.sun.star.style.DropCapFormat specifies whether the first characters of the paragraph are displayed in capital letters and how they are formatted.
|
DropCapWholeWord | [optional] boolean - Specifies if the property DropCapFormat is applied to the whole first word.
|
ParaKeepTogether | [optional] boolean - Setting this property to true prevents page or column breaks between this and the following paragraph.
|
ParaSplit | [optional] boolean - Setting this property to false prevents the paragraph from getting split into two pages or columns.
|
NumberingLevel | [optional] short - Specifies the numbering level of the paragraph.
|
NumberingRules | com.sun.star.container.XIndexReplace. Contains the numbering rules applied to this paragraph. |
NumberingStartValue | [optional] short - Specifies the start value for numbering if a new numbering starts at this paragraph.
|
ParaIsNumberingRestart | [optional] boolean - Determines if the numbering rules restart, counting at the current paragraph.
|
NumberingStyleName | [optional] string - Specifies the name of the style for the numbering.
|
ParaOrphans | [optional] byte - Specifies the minimum number of lines of the paragraph that have to be at bottom of a page if the paragraph is spread over more than one page.
|
ParaWidows | [optional] byte - Specifies the minimum number of lines of the paragraph that have to be at top of a page if the paragraph is spread over more than one page.
|
ParaShadowFormat | [optional] struct com.sun.star.table.ShadowFormat. Determines the type, color, and size of the shadow.
|
ParaIsHangingPunctuation | [optional] boolean - Determines if hanging punctuation is allowed.
|
ParaIsCharacterDistance | [optional] boolean - Determines if a distance between Asian text, western text or complex text is set.
|
ParaIsForbiddenRules | [optional] boolean - Determines if the the rules for forbidden characters at the start or end of text lines are considered.
|
com.sun.star.style.ParagraphPropertiesAsian describes some further properties used in Asian text.
Properties of com.sun.star.style.ParagraphPropertiesAsian | |
---|---|
ParaIsHangingPunctuation | [optional] boolean - Determines if hanging punctuation is allowed.
|
ParaIsCharacterDistance | [optional] boolean - Determines if a distance between Asian text, western text or complex text is set.
|
ParaIsForbiddenRules | [optional] boolean - Determines if the the rules for forbidden characters at the start or end of text lines are considered.
|
Objects supporting these properties support com.sun.star.beans.XPropertySet, as well. To change the properties, use the method setPropertyValue()
.
/** This snippet shows the necessary steps to set a property at the
current position of a given text cursor mxDocCursor
*/
// query the XPropertySet interface
XPropertySet xCursorProps = UnoRuntime.queryInterface(XPropertySet.class, mxDocCursor);
// call setPropertyValue, passing in a Float object
xCursorProps.setPropertyValue("CharWeight", new Float ( com.sun.star.awt.FontWeight.BOLD));
The same procedure is used for all properties. The more complex properties are described here.
If a change of the page style is required the paragraph property PageDescName
has to be set using an existing page style name. This forces a page break at the cursor position and the new inserted page uses the requested page style. The property PageNumberOffset
has to be set to start with a new page count. If inserting an additional paragraph should be avoided, the cursor must be placed at the beginning of the first paragraph before inserting it.
If a page break (or a column break) without a change in the used style is required, the property BreakType
is set using the values of com.sun.star.style.BreakType:
Page break | Description |
---|---|
BreakType | Page or column break as described in com.sun.star.style.BreakType. Possible values are NONE, COLUMN_BEFORE, COLUMN_AFTER, COLUMN_BOTH, PAGE_BEFORE, PAGE_AFTER, and PAGE_BOTH. Setting the property forces a page or column break at the current text cursor position, paragraph or text table. |
The property ParaLineNumberCount
is used to include a paragraph in the line numbering. The setting of the line numbering options is done using the property set provided by the com.sun.star.text.XLineNumberingProperties interface implemented at the text document model.
To create a hyperlink these properties are set at the current cursor position or the current com.sun.star.text.Paragraph service.
Hyperlink properties are not specified for paragraphs in the API reference.
Hyperlink Properties | Description |
---|---|
HyperLinkURL
|
string - Contains the URL.
|
HyperLinkTarget
|
string - Contains the name of the target frame and can be left blank.
|
HyperLinkName
|
string - The name of the hyperlink can be left blank.
|
UnvisitedCharStyleName
|
string - The names of the character styles used to emphasize visited or not visited links. If left blank, the default character styles Internet Link/Visited Internet Link are applied automatically.
|
VisitedCharStyleName
| |
HyperLinkEvents
|
Events attached to the hyperlink. The names of the events are OnClick, OnMouseOver, and OnMouseOut. Each returned event is a sequence of com.sun.star.beans.PropertyValue, with three elements named EventType, MacroName and Library. All elements contain string values. The EventType contains the value "StarBasic" for LibreOffice Basic macros . The macro name contains the path to the macro, for example, Standard.Module1.Main. The library contains the name of the library. |
Some properties are connected with each other. There may be side effects or dependencies between the following properties:
Interdependencies between Properties |
---|
ParaRightMargin , ParaLeftMargin , ParaFirstLineIndent , ParaIsAutoFirstLineIndent
|
ParaTopMargin , ParaBottomMargin
|
ParaGraphicURL/Filter/Location , ParaBackColor , ParaBackTransparent
|
ParaIsHyphenation , ParaHyphenationMaxLeadingChars/MaxTrailingChars/MaxHyphens
|
Left/Right/Top/BottomBorder , Left/Right/Top/BottomBorderDistance , BorderDistance
|
DropCapFormat , DropCapWholeWord , DropCapCharStyleName
|
PageDescName , PageNumberOffset , PageStyleName
|
HyperLinkURL/Name/Target , UnvisitedCharStyleName , VisitedCharStyleName
|
CharEscapement , CharAutoEscapement , CharEscapementHeight
|
CharFontName , CharFontStyleName , CharFontFamily , CharFontPitch
|
CharStrikeOut , CharCrossedOut
|
CharUnderline , CharUnderlineColor , CharUnderlineHasColor
|
CharCombineIsOn , CharCombinePrefix , CharCombineSuffix
|
RubyText , RubyAdjust , RubyCharStyleName , RubyIsAbove
|
Cursors
The text model cursor allows for free navigation over the model by character, words, sentences, or paragraphs. There can be several model cursors at the same time. Model cursor creation, movement and usage is discussed in the section Word Processing . The text model cursors are com.sun.star.text.TextCursor services that are based on the interface com.sun.star.text.XTextCursor, which is based on com.sun.star.text.XTextRange.
The text view cursor enables the user to travel over the document in the view by character, line, screen page and document page. There is only one text view cursor. Certain information about the current layout, such as the number of lines and page number must be retrieved at the view cursor. The chapter Text Document Controller below discusses the view cursor in detail. The text view cursor is a com.sun.star.text.TextViewCursor service that includes com.sun.star.text.TextLayoutCursor.
Locating Text Contents
The text document model has suppliers that yield all text contents in a document as collections. To find a particular text content, such as bookmarks or text fields, use the appropriate supplier interface. The following supplier interfaces are available at the model:
Supplier interfaces | Methods |
---|---|
com.sun.star.text.XTextTablesSupplier | com.sun.star.container.XNameAccess getTextTables()
|
com.sun.star.text.XTextFramesSupplier | com.sun.star.container.XNameAccess getTextFrames()
|
com.sun.star.text.XTextGraphicObjectsSupplier | com.sun.star.container.XNameAccess getGraphicObjects()
|
com.sun.star.text.XTextEmbeddedObjectsSupplier | com.sun.star.container.XNameAccess getEmbeddedObjects()
|
com.sun.star.text.XTextFieldsSupplier | com.sun.star.container.XEnumerationAccess getTextFields()
|
com.sun.star.text.XBookmarksSupplier | com.sun.star.container.XNameAccess getBookmarks()
|
com.sun.star.text.XReferenceMarksSupplier | com.sun.star.container.XNameAccess getReferenceMarks()
|
com.sun.star.text.XFootnotesSupplier | com.sun.star.container.XIndexAccess getFootnotes() com.sun.star.beans.XPropertySet getFootnoteSettings()
|
com.sun.star.text.XEndnotesSupplier | com.sun.star.container.XIndexAccess getEndnotes()
|
com.sun.star.text.XTextSectionsSupplier | com.sun.star.container.XNameAccess getTextSections()
|
com.sun.star.text.XDocumentIndexesSupplier | com.sun.star.container.XIndexAccess getDocumentIndexes()
|
com.sun.star.document.XRedlinesSupplier | com.sun.star.container.XEnumerationAccess getRedlines()
|
You can work with text content directly, set properties and use its interfaces, or find out where it is and do an action at the text content location in the text. To find out where a text content is located call the getAnchor()
method at the interface com.sun.star.text.XTextContent, which every text content must support.
In addition, text contents located at the current text cursor position or the content where the cursor is currently located are provided in the PropertySet
of the cursor. The corresponding cursor properties are:
DocumentIndexMark
TextField
ReferenceMark
Footnote
Endnote
DocumentIndex
TextTable
TextFrame
Cell
TextSection
Search and Replace
The writer model supports the interface com.sun.star.util.XReplaceable that inherits from the interface com.sun.star.util.XSearchable for searching and replacing in text. It contains the following methods:
com::sun::star::util::XSearchDescriptor createSearchDescriptor()
com::sun::star::util::XReplaceDescriptor createReplaceDescriptor()
com::sun::star::uno::XInterface findFirst( [in] com::sun::star::util::XSearchDescriptor xDesc)
com::sun::star::uno::XInterface findNext( [in] com::sun::star::uno::XInterface xStartAt,
[in] com::sun::star::util::XSearchDescriptor xDesc)
com::sun::star::container::XIndexAccess findAll( [in] com::sun::star::util::XSearchDescriptor xDesc)
long replaceAll( [in] com::sun::star::util::XSearchDescriptor xDesc)
To search or replace text, first create a descriptor service using createSearchDescriptor()
or createReplaceDescriptor()
. You receive a service that supports the interface com.sun.star.util.XPropertyReplace with methods to describe what you are searching for, what you want to replace with and what attributes you are looking for. It is described in detail below.
Pass in this descriptor to the methods findFirst()
, findNext()
, findAll()
or replaceAll()
.
The methods findFirst()
and findNext()
return a com.sun.star.uno.XInterface pointing to an object that contains the found item. If the search is not successful, a null reference to an XInterface
is returned, that is, if you try to query other interfaces from it, null is returned. The method findAll()
returns a com.sun.star.container.XIndexAccess containing one or more com.sun.star.uno.XInterface pointing to the found text ranges or if they failed an empty interface. The method replaceAll()
returns the number of replaced occurrences only.
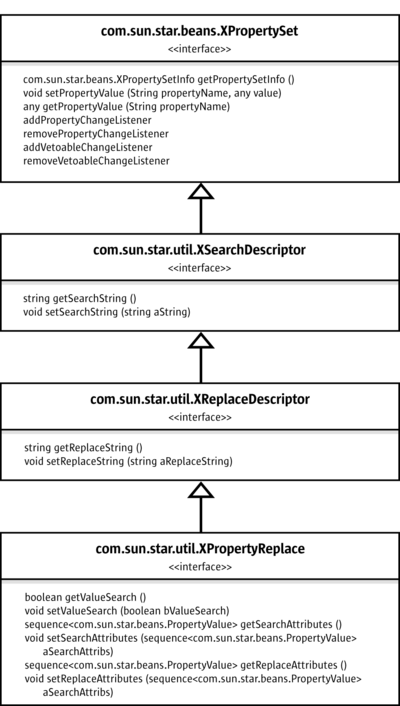
The interface com.sun.star.util.XPropertyReplace is required to describe your search. It is a powerful interface and inherits from XReplaceDescriptor
, XSearchDescriptor
and XPropertySet
.
The target of your search is described by a string containing a search text or a style name using setSearchString()
. Correspondingly, provide the text string or style name that should replace the found occurrence of the search target to the XReplaceDescriptor
using setReplaceString()
. Refine the search mode through the properties included in the service com.sun.star.util.SearchDescriptor:
Properties of com.sun.star.util.SearchDescriptor | |
---|---|
com.sun.star.util.SearchDescriptor.SearchBackwards | boolean - Search backward
|
com.sun.star.util.SearchDescriptor.SearchCaseSensitive | boolean - Search is case sensitive.
|
com.sun.star.util.SearchDescriptor.SearchRegularExpression | boolean - Search interpreting the search string as a regular expression.
|
com.sun.star.util.SearchDescriptor.SearchSimilarity | boolean - Use similarity search using the four following options:
|
com.sun.star.util.SearchDescriptor.SearchSimilarityAdd | short - Determines the number of characters the word in the document may be longer than the search string for it to remain valid.
|
com.sun.star.util.SearchDescriptor.SearchSimilarityExchange | short - Determines how many characters in the search term can be exchanged.
|
com.sun.star.util.SearchDescriptor.SearchSimilarityRelax | boolean - If true , the values of added, exchanged, and removed characters are combined The search term is then found if the word in the document can be generated through any combination of these three conditions.
|
com.sun.star.util.SearchDescriptor.SearchSimilarityRemove | short - Determines how many characters the word in the document may be shorter than the search string for it to remain valid. The characters may be removed from the word at any position.
|
com.sun.star.util.SearchDescriptor.SearchStyles | boolean - Determines if the search and replace string should be interpreted as paragraph style names. Note that the Display Name of the style has to be used.
|
com.sun.star.util.SearchDescriptor.SearchWords | boolean - Determines if the search should find complete words only.
|
SearchType (not included in API-reference ) | integer - Determines if the search should be done in cell formulas (0) values (1) or notes (2). [Calc only]
|
In XPropertyReplace
, the methods to get and set search attributes, and replace attributes allow the attributes to search for to be defined and the attributes to insert instead of the existing attributes. All of these methods expect a sequence of com.sun.star.beans.PropertyValue structs.
Any properties contained in the services com.sun.star.style.CharacterProperties, com.sun.star.style.CharacterPropertiesAsian and com.sun.star.style.ParagraphProperties can be used for an attribute search. If setValueSearch(false)
is used, LibreOffice checks if an attribute exists, whereas setValueSearch(true)
finds specific attribute values. If only searching to see if an attribute exists at all, it is sufficient to pass a PropertyValue
struct with the Name field set to the name of the required attribute.
The following code snippet replaces all occurrences of the text "random numbers" by the bold text "replaced numbers" in a given document mxDoc
.
XReplaceable xReplaceable = UnoRuntime.queryInterface(XReplaceable.class, mxDoc);
XReplaceDescriptor xRepDesc = xReplaceable.createReplaceDescriptor();
// set a string to search for
xRepDesc.setSearchString("random numbers");
// set the string to be inserted
xRepDesc.setReplaceString("replaced numbers");
// create an array of one property value for a CharWeight property
PropertyValue[] aReplaceArgs = new PropertyValue[1];
// create PropertyValue struct
aReplaceArgs[0] = new PropertyValue();
// CharWeight should be bold
aReplaceArgs[0].Name = "CharWeight";
aReplaceArgs[0].Value = new Float(com.sun.star.awt.FontWeight.BOLD);
// set our sequence with one property value as ReplaceAttribute
XPropertyReplace xPropRepl = UnoRuntime.queryInterface(
XPropertyReplace.class, xRepDesc);
xPropRepl.setReplaceAttributes(aReplaceArgs);
// replace
long nResult = xReplaceable.replaceAll(xRepDesc);
Tables
Table Architecture
LibreOffice text tables consist of rows, rows consist of one or more cells, and cells can contain text or rows. There is no logical concept of columns. From the API's perspective, a table acts as if it had columns, as long as there are no split or merged cells.
Cells in a row are counted alphabetically starting from A, where rows are counted numerically, starting from 1. This results in a cell-row addressing pattern, where the cell letter is denoted first (A-Zff.), followed by the row number (1ff.):
A1 | B1 | C1 | D1 |
A2 | B2 | C2 | D2 |
A3 | B3 | C3 | D3 |
A4 | B4 | C4 | D4 |
When a cell is split vertically, the new cell gets the letter of the former right-hand-side neighbor cell and the former neighbor cell gets the next letter in the alphabet. Consider the example table below: B2 was split vertically, a new cell C2 is inserted and the former C2 became D2, D2 became E2, and so forth.
When cells are merged vertically, the resulting cell counts as one cell and gets one letter. The neighbor cell to the right gets the subsequent letter. B4 in the table below shows this. The former B4 and C4 have been merged, so the former D4 could become C4. The cell name D4 is no longer required.
As shown, there is no way to address a column C anymore, for the cells C1 to C4 no longer form a column:
A1 | B1 | C1 | D1 | |
A2 | B2 vertically split in two | C2 newly inserted | D2 | E2 |
A3 | B3 | C3 | D3 | |
A4 | B4 merged with C4 | C4 |
When cells are split horizontally, LibreOffice simply inserts as many rows into the cell as required.
In our example table, we continued by splitting C2 first horizontally and then vertically so that there is a range of four cells.
The writer treats the content of C2 as two rows and starts counting cells within rows. To address the new cells, it extends the original cell name C2 by new addresses following the cell-row pattern. The upper row gets row number 1 and the first cell in the row gets cell number 1, resulting in the cell address C2.1.1, where the latter 1 indicates the row and the former 1 indicates the first cell in the row. The right neighbor of C2.1.1 is C2.2.1. The subaddress 2.1 means the second cell in the first row.
A1 | B1 | C1 | D1 | ||
A2 | B2 vertically split in two | C2.1.1 | C2.2.1 | D2 | E2 |
C2.1.2 | C2.2.2 | ||||
A3 | B3 | C3 | D3 | ||
A4 | B4 merged with C4 | C4 |
The cell-row pattern is used for all further subaddressing as the cells are split and merged. The cell addresses can change radically depending on the table structure generated by LibreOffice. The next table shows what happens when E2 is merged with D3. The table is reorganized, so that it has three rows instead of four. The second row contains two cells, A2 and B2 (sic!). The cell A2 has two rows, as shown from the cell subaddresses: The upper row consists of four cells, namely A2.1.1 through A2.4.1, whereas the lower row consists of the three cells A2.1.2 through A2.3.2.
The cell range C2.1.1:C2.2.2 that was formerly contained in cell C2 is now in cell A2.3.1 that denotes the third cell in the first row of A2. Within the address of the cell A2.3.1, LibreOffice has started a new subaddressing level using the cell-row pattern again.
A1 | B1 | C1 | D1 | ||
A2.1.1 | A2.2.1 | A2.3.1.1.1 | A2.3.1.2.1 | A2.4.1 | Former E2 merged
with former D3 Becomes B2! |
A2.3.1.1.2 | A2.3.1.2.2 | ||||
A2.1.2 | A2.2.2 | A2.3.2 | |||
A3 | B3 | C3 |
Cell addresses can become complicated. The cell address can be looked up in the user interface. Set the GUI text cursor in the desired cell and observe the lower-right corner of the status bar in the text document.
Remember that there are only "columns" in a text table, as long as there are no split or merged cells.
Text tables support the service com.sun.star.text.TextTable, which includes the service com.sun.star.text.TextContent:
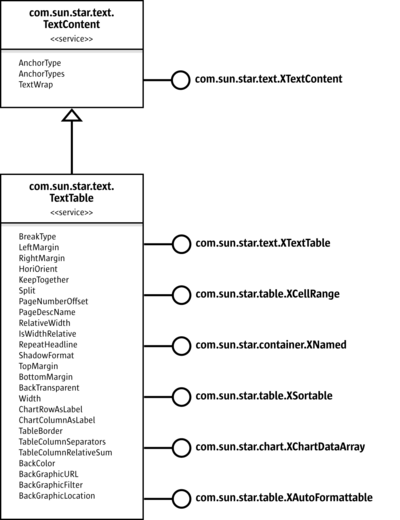
The service com.sun.star.text.TextTable offers access to table cells in two different ways::
- Yields named table cells which are organized in rows and columns.
- Provides a table cursor to travel through the table cells and alter the cell properties.
These aspects are reflected in the interface com.sun.star.text.XTextTable which inherits from com.sun.star.text.XTextContent. It can be seen as a rectangular range of cells defined by numeric column indexes, as described by com.sun.star.table.XCellRange. This aspect makes text tables compatible with spreadsheet tables. Also, text tables have a name, can be sorted, charts can be based on them, and predefined formats can be applied to the tables. The latter aspects are covered by the interfaces com.sun.star.container.XNamed, com.sun.star.util.XSortable, com.sun.star.chart.XChartDataArray and com.sun.star.table.XAutoFormattable.
The usage of these interfaces and the properties of the TextTable service are discussed below.
Named Table Cells in Rows, Columns and the Table Cursor
The interface XTextTable
introduces the following methods to initialize a table, work with table cells, rows and columns, and create a table cursor:
void initialize( [in] long nRows, [in] long nColumns)
sequence< string > getCellNames() com::sun::star::table::XCell getCellByName( [in] string aCellName)
com::sun::star::table::XTableRows getRows() com::sun::star::table::XTableColumns getColumns()
com::sun::star::text::XTextTableCursor createCursorByCellName( [in] string aCellName)
The method initialize()
sets the number of rows and columns prior to inserting the table into the text. Non-initialized tables default to two rows and two columns.
The method getCellNames()
returns a sequence of strings containing the names of all cells in the table in A1[.1.1] notation.
The method getCellByName()
expects a cell name in A1[.1.1] notation, and returns a cell object that is a com.sun.star.table.XCell and a com.sun.star.text.XText. The advantage of getCellByName()
is its ability to retrieve cells even in tables with split or merged cells.
The method getRows()
returns a table row container supporting com.sun.star.table.XTableRows that is a com.sun.star.container.XIndexAccess, and introduces the following methods to insert an arbitrary number of table rows below a given row index position and remove rows from a certain position:
void insertByIndex ( [in] long nIndex, [in] long nCount) void removeByIndex ( [in] long nIndex, [in] long nCount)
The following table shows which XTableRows
methods work under which circumstances.
Method in com.sun.star.table.XTableRows | In Simple table | In Complex Table |
---|---|---|
getElementType() | X | X |
hasElements() | X | X |
getByIndex() | X | X |
getCount() | X | X |
insertByIndex() | X | - |
removeByIndex() | X | - |
Every row returned by getRows()
supports the service com.sun.star.text.TextTableRow, that is, it is a com.sun.star.beans.XPropertySet which features these properties:
Properties of com.sun.star.text.TextTableRow | |
---|---|
BackColor | long - Specifies the color of the background in 0xAARRGGBB notation.
|
BackTransparent | boolean - If true, the background color value in "BackColor " is not visible.
|
BackGraphicURLBackGraphicURL | string - Contains the URL of a background graphic.
|
BackGraphicFilter | string - Contains the name of the file filter of a background graphic.
|
BackGraphicLocation | com.sun.star.style.GraphicLocation. Determines the position of the background graphic. |
TableColumnSeparators | Defines the column width and its merging behavior. It contains a sequence of com.sun.star.text.TableColumnSeparator structs with the fields Position and IsVisible. The value of Position is relative to the table property TableColumnRelativeSum. IsVisible refers to merged cells where the separator becomes invisible. |
Height | long - Contains the height of the table row.
|
IsAutoHeight | boolean - If the value of this property is true , the height of the table row depends on the content of the table cells.
|
The method getColumns()
is similar to getRows()
, but restrictions apply. It returns a table column container supporting com.sun.star.table.XTableColumns that is a com.sun.star.container.XIndexAccess and introduces the following methods to insert an arbitrary number of table columns behind a given column index position and remove columns from a certain position:
void insertByIndex( [in] long nIndex, [in] long nCount) void removeByIndex( [in] long nIndex, [in] long nCount)
The following table shows which XTableColumns
methods work in which situation.
Methods in com.sun.star.table.XTableColumns | In Simple Table | In Complex Table |
---|---|---|
getElementType() | X | X |
hasElements() | X | X |
getByIndex() | X (but returned object supports XInterface only) | - |
getCount() | X | - |
insertByIndex() | X | - |
removeByIndex() | X | - |
The method createCursorByCellName()
creates a text table cursor that can select a cell range in the table, merge or split cells, and read and write cell properties of the selected cell range. It is a com.sun.star.text.TextTableCursor service with the interfaces com.sun.star.text.XTextTableCursor and com.sun.star.beans.XPropertySet.
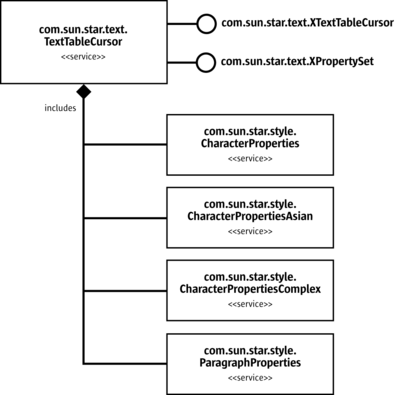
These are the methods contained in XTextTableCursor
:
string getRangeName()
boolean goLeft( [in] short nCount, [in] boolean bExpand) boolean goRight( [in] short nCount, [in] boolean bExpand) boolean goUp( [in] short nCount, [in] boolean bExpand) boolean goDown( [in] short nCount, [in] boolean bExpand)
void gotoStart( [in] boolean bExpand) void gotoEnd( [in] boolean bExpand) boolean gotoCellByName( [in] string aCellName, [in] boolean bExpand)
boolean mergeRange() boolean splitRange( [in] short Count, [in] boolean Horizontal)
Traveling through the table calls the cursor's goLeft()
, goRight()
, goUp()
, goDown()
, gotoStart()
, gotoEnd()
, and gotoCellByName()
methods, passing true to select cells on the way.
Once a cell range is selected, apply character and paragraph properties to the cells in the range as defined in the services com.sun.star.style.CharacterProperties, com.sun.star.style.CharacterPropertiesAsian, com.sun.star.style.CharacterPropertiesComplex and com.sun.star.style.ParagraphProperties. Moreover, split and merge cells using the text table cursor. An example is provided below.
Indexed Cells and Cell Ranges
The interface com.sun.star.table.XCellRange provides access to cells using their row and column index as position, and to create sub ranges of tables:
com::sun::star::table::XCell getCellByPosition( [in] long nColumn, [in] long nRow) com::sun::star::table::XCellRange getCellRangeByPosition( [in] long nLeft, [in] long nTop, [in] long nRight, [in] long nBottom) com::sun::star::table::XCellRange getCellRangeByName( [in] string aRange)
The method getCellByPosition()
returns a cell object supporting the interfaces com.sun.star.table.XCell and com.sun.star.text.XText. To find the cell the name is internally created from the position using the naming scheme described above and returns this cell if it exists. Calling getCellByPosition(1, 1)
in the table at the beginning of this chapter returns the cell "B2" .
The methods getCellRangeByPosition()
and getCellRangeByName()
return a range object that is described below. The name of the range is created with the top-left cell and bottom-right cell of the table separated by a colon :
as in A1:B4
. Both methods fail when the structure of the table contains merged or split cells.
Table Naming, Sorting, Charting and Autoformatting
Each table has a unique name that can be read and written using the interface com.sun.star.container.XNamed.
A text table is a XNamed. Its method createSortDescriptor()
returns a sequence of com.sun.star.beans.PropertyValue structs that provides the elements as described in the service com.sun.star.text.TextSortDescriptor. The method sort()
sorts the table content by the given parameters.
The interface com.sun.star.chart.XChartDataArray is used to connect a table or a range inside of a table to a chart. It reads and writes the values of a range, and sets the column and row labels. The inherited interface com.sun.star.chart.XChartData enables the chart to connect listeners to be notified when changes to the values of a table are made. For details about charting, refer to chapter Charts.
The interface com.sun.star.table.XAutoFormattable provides in its method autoFormat()
a method to format the table using a predefined table format. To access the available auto formats, the service com.sun.star.sheet.TableAutoFormats has to be accessed. For details, refer to chapter Table Auto Formats.
Text Table Properties
The text table supports the properties described in the service com.sun.star.text.TextTable:
Properties of com.sun.star.text.TextTable | |
---|---|
BackColor | long - Contains the color of the table background.
|
BackGraphicFilter | string - Contains the name of the file filter for the background graphic.
|
BackGraphicLocation | com.sun.star.style.GraphicLocation. Determines the position of the background graphic. |
BackGraphicURL | string - Contains the URL for the background graphic.
|
BackTransparent | boolean - Determines if the background color is transparent.
|
BottomMargin | long - Determines the bottom margin.
|
BreakType | com.sun.star.style.BreakType. Determines the type of break that is applied at the beginning of the table. |
ChartColumnAsLabel | boolean - Determines if the first column of the table should be treated as axis labels when a chart is to be created.
|
ChartRowAsLabel | boolean - Determines if the first row of the table should be treated as axis labels when a chart is to be created.
|
HoriOrient | short - Contains the horizontal orientation according to com.sun.star.text.HoriOrientation.
|
IsWidthRelative | boolean - Determines if the value of the relative width is valid.
|
KeepTogether | boolean - Setting this property to true prevents page or column breaks between this table and the following paragraph or text table.
|
LeftMargin | long - Contains the left margin of the table.
|
PageDescName | string - If this property is set, it creates a page break before the table and assigns the value as the name of the new page style sheet to use.
|
PageNumberOffset | short - If a page break property is set at the table, this property contains the new value for the page number.
|
RelativeWidth | short - Determines the width of the table relative to its environment.
|
RepeatHeadline | boolean - Determines if the first row of the table is repeated on every new page.
|
RightMargin | long - Contains the right margin of the table.
|
ShadowFormat | struct com.sun.star.table.ShadowFormat determines the type, color and size of the shadow.
|
Split | boolean - Setting this property to false prevents the table from getting spread on two pages.
|
TableBorder | struct com.sun.star.table.TableBorder. Contains the description of the table borders. |
TableColumnRelativeSum | short - Contains the sum of the column width values used in TableColumnSeparators.
|
TableColumnSeparators | sequence < com.sun.star.text.TableColumnSeparator >. Defines the column width and its merging behavior. It contains a sequence of com.sun.star.text.TableColumnSeparator structs with the fields Position and IsVisible. The value of Position is relative to the table property TableColumnRelativeSum. IsVisible refers to merged cells where the separator becomes invisible. In tables with merged or split cells, the sequence TableColumnSeparators is empty.
|
TopMargin | long - Determines the top margin.
|
Width | long - Contains the absolute table width.
|
Inserting Tables
To create and insert a new text table, a five-step procedure must be followed:
- Get the service manager of the text document, querying the document's factory interface com.sun.star.lang.XMultiServiceFactory.
- Order a new text table from the factory by its service name "
com.sun.star.text.TextTable
", using the factory methodcreateInstance()
. - From the object received, query the com.sun.star.text.XTextTable interface that inherits from com.sun.star.text.XTextContent.
- If necessary, initialize the table with the number of rows and columns. For this purpose,
XTextTable
offers theinitialize()
method. - Insert the table into the text using the
insertTextContent()
method at its com.sun.star.text.XText interface. The methodinsertTextContent()
expects anXTextContent
to insert. SinceXTextTable
inherits fromXTextContent
, pass theXTextTable
interface retrieved previously.
You are now ready to get cells, fill in text, values and formulas and set the table and cell properties as needed.
In the following code sample, there is a small helper function to put random numbers between -1000 and 1000 into the table to demonstrate formulas:
/** This method returns a random double which isn't too high or too low
*/
protected double getRandomDouble()
{
return (maRandom.nextInt(1000) * maRandom.nextDouble());
}
The following helper function inserts a string into a cell known by its name and sets its text color to white:
/** This method sets the text colour of the cell refered to by sCellName to white and inserts
the string sText in it
*/
public static void insertIntoCell(String sCellName, String sText, XTextTable xTable) {
// Access the XText interface of the cell referred to by sCellName
XText xCellText = UnoRuntime.queryInterface(
XText.class, xTable.getCellByName(sCellName));
// create a text cursor from the cells XText interface
XTextCursor xCellCursor = xCellText.createTextCursor();
// Get the property set of the cell's TextCursor
XPropertySet xCellCursorProps = UnoRuntime.queryInterface(
XPropertySet.class, xCellCursor);
try {
// Set the colour of the text to white
xCellCursorProps.setPropertyValue("CharColor", Integer.valueOf(16777215));
} catch (Exception e) {
e.printStackTrace(System.err);
}
// Set the text in the cell to sText
xCellText.setString(sText);
}
Using the above helper functions, create a text table and insert it into the text document.
/** This method shows how to create and insert a text table, as well as insert text and formulae
into the cells of the table
*/
protected void TextTableExample ()
{
try
{
// Create a new table from the document's factory
XTextTable xTable = UnoRuntime.queryInterface(
XTextTable.class, mxDocFactory .createInstance(
"com.sun.star.text.TextTable" ) );
// Specify that we want the table to have 4 rows and 4 columns
xTable.initialize( 4, 4 );
// Insert the table into the document
mxDocText.insertTextContent( mxDocCursor, xTable, false);
// Get an XIndexAccess of the table rows
XIndexAccess xRows = xTable.getRows();
// Access the property set of the first row (properties listed in service description:
// com.sun.star.text.TextTableRow)
XPropertySet xRow = UnoRuntime.queryInterface(
XPropertySet.class, xRows.getByIndex ( 0 ) );
// If BackTransparant is false, then the background color is visible
xRow.setPropertyValue( "BackTransparent", Boolean.FALSE);
// Specify the color of the background to be dark blue
xRow.setPropertyValue( "BackColor", Integer.valueOf(6710932));
// Access the property set of the whole table
XPropertySet xTableProps = UnoRuntime.queryInterface(
XPropertySet.class, xTable );
// We want visible background colors
xTableProps.setPropertyValue( "BackTransparent", Boolean.FALSE);
// Set the background colour to light blue
xTableProps.setPropertyValue( "BackColor", Integer.valueOf(13421823));
// set the text (and text colour) of all the cells in the first row of the table
insertIntoCell( "A1", "First Column", xTable );
insertIntoCell( "B1", "Second Column", xTable );
insertIntoCell( "C1", "Third Column", xTable );
insertIntoCell( "D1", "Results", xTable );
// Insert random numbers into the first this three cells of each
// remaining row
xTable.getCellByName( "A2" ).setValue( getRandomDouble() );
xTable.getCellByName( "B2" ).setValue( getRandomDouble() );
xTable.getCellByName( "C2" ).setValue( getRandomDouble() );
xTable.getCellByName( "A3" ).setValue( getRandomDouble() );
xTable.getCellByName( "B3" ).setValue( getRandomDouble() );
xTable.getCellByName( "C3" ).setValue( getRandomDouble() );
xTable.getCellByName( "A4" ).setValue( getRandomDouble() );
xTable.getCellByName( "B4" ).setValue( getRandomDouble() );
xTable.getCellByName( "C4" ).setValue( getRandomDouble() );
// Set the last cell in each row to be a formula that calculates
// the sum of the first three cells
xTable.getCellByName( "D2" ).setFormula( "sum <A2:C2>" );
xTable.getCellByName( "D3" ).setFormula( "sum <A3:C3>" );
xTable.getCellByName( "D4" ).setFormula( "sum <A4:C4>" );
}
catch (Exception e)
{
e.printStackTrace ( System.out );
}
}
The next sample inserts auto text entries into a table, splitting cells during its course.
/** This example demonstrates the use of the AutoTextContainer, AutoTextGroup and AutoTextEntry services
and shows how to create, insert and modify auto text blocks
*/
protected void AutoTextExample ()
{
try
{
// Go to the end of the document
mxDocCursor.gotoEnd( false );
// Insert two paragraphs
mxDocText.insertControlCharacter ( mxDocCursor,
ControlCharacter.PARAGRAPH_BREAK, false );
mxDocText.insertControlCharacter ( mxDocCursor,
ControlCharacter.PARAGRAPH_BREAK, false );
// Position the cursor in the second paragraph
XParagraphCursor xParaCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, mxDocCursor );
xParaCursor.gotoPreviousParagraph ( false );
// Get an XNameAccess interface to all auto text groups from the document factory
XNameAccess xContainer = UnoRuntime.queryInterface(
XNameAccess.class, mxFactory.createInstance (
"com.sun.star.text.AutoTextContainer" ) );
// Create a new table at the document factory
XTextTable xTable = UnoRuntime.queryInterface(
XTextTable.class, mxDocFactory .createInstance(
"com.sun.star.text.TextTable" ) );
// Store the names of all auto text groups in an array of strings
String[] aGroupNames = xContainer.getElementNames();
// Make sure we have at least one group name
if ( aGroupNames.length > 0 )
{
// initialise the table to have a row for every autotext group
//in a single column + one
// additional row for a header
xTable.initialize( aGroupNames.length+1,1);
// Access the XPropertySet of the table
XPropertySet xTableProps = UnoRuntime.queryInterface(
XPropertySet.class, xTable );
// We want a visible background
xTableProps.setPropertyValue( "BackTransparent", Boolean.FALSE);
// We want the background to be light blue
xTableProps.setPropertyValue( "BackColor", Integer.valueOf(13421823));
// Insert the table into the document
mxDocText.insertTextContent( mxDocCursor, xTable, false);
// Get an XIndexAccess to all table rows
XIndexAccess xRows = xTable.getRows();
// Get the first row in the table
XPropertySet xRow = UnoRuntime.queryInterface(
XPropertySet.class, xRows.getByIndex ( 0 ) );
// We want the background of the first row to be visible too
xRow.setPropertyValue( "BackTransparent", Boolean.FALSE);
// And let's make it dark blue
xRow.setPropertyValue( "BackColor", Integer.valueOf(6710932));
// Put a description of the table contents into the first cell
insertIntoCell( "A1", "AutoText Groups", xTable);
// Create a table cursor pointing at the second cell in the first column
XTextTableCursor xTableCursor = xTable.createCursorByCellName ( "A2" );
// Loop over the group names
for ( int i = 0 ; i < aGroupNames.length ; i ++ )
{
// Get the name of the current cell
String sCellName = xTableCursor.getRangeName ();
// Get the XText interface of the current cell
XText xCellText = UnoRuntime.queryInterface (
XText.class, xTable.getCellByName ( sCellName ) );
// Set the cell contents of the current cell to be
// the name of the autotext group
xCellText.setString ( aGroupNames[i] );
// Access the autotext group with this name
XAutoTextGroup xGroup = UnoRuntime.queryInterface (
XAutoTextGroup.class,xContainer.getByName(aGroupNames[i]));
// Get the titles of each autotext block in this group
String [] aBlockNames = xGroup.getTitles();
// Make sure that the autotext group contains at least one block
if ( aBlockNames.length > 0 )
{
// Split the current cell vertically into two seperate cells
xTableCursor.splitRange ( (short) 1, false );
// Put the cursor in the newly created right hand cell
// and select it
xTableCursor.goRight ( (short) 1, false );
// Split this cell horizontally to make a seperate cell
// for each Autotext block
if ( ( aBlockNames.length -1 ) > 0 )
xTableCursor.splitRange (
(short) (aBlockNames.length - 1), true );
// loop over the block names
for ( int j = 0 ; j < aBlockNames.length ; j ++ )
{
// Get the XText interface of the current cell
xCellText = UnoRuntime.queryInterface (
XText.class, xTable.getCellByName (
xTableCursor.getRangeName() ) );
// Set the text contents of the current cell to the
// title of an Autotext block
xCellText.setString ( aBlockNames[j] );
// Move the cursor down one cell
xTableCursor.goDown( (short)1, false);
}
}
// Go back to the cell we originally split
xTableCursor.gotoCellByName ( sCellName, false );
// Go down one cell
xTableCursor.goDown( (short)1, false);
}
XAutoTextGroup xGroup;
String [] aBlockNames;
// Add a depth so that we only generate 200 numbers before
// giving up on finding a random autotext group that contains autotext blocks
int nDepth = 0;
do
{
// Generate a random, positive number which is lower than
// the number of autotext groups
int nRandom = maRandom.nextInt( aGroupNames.length );
// Get the autotext group at this name
xGroup = UnoRuntime.queryInterface (
XAutoTextGroup.class, xContainer.getByName (
aGroupNames[ nRandom ] ) );
// Fill our string array with the names of all the blocks in this
// group
aBlockNames = xGroup.getElementNames();
// increment our depth counter
++nDepth;
}
while ( nDepth < 200 && aBlockNames.length == 0 );
// If we managed to find a group containg blocks...
if ( aBlockNames.length > 0 )
{
// Pick a random block in this group and get it's
// XAutoTextEntry interface
int nRandom = maRandom.nextInt( aBlockNames.length );
XAutoTextEntry xEntry = ( XAutoTextEntry )
UnoRuntime.queryInterface (
XAutoTextEntry.class, xGroup.getByName (
aBlockNames[ nRandom ] ) );
// insert the modified autotext block at the end of the document
xEntry.applyTo ( mxDocCursor );
// Get the titles of all text blocks in this AutoText group
String [] aBlockTitles = xGroup.getTitles();
// Get the XNamed interface of the autotext group
XNamed xGroupNamed = UnoRuntime.queryInterface (
XNamed.class, xGroup );
// Output the short cut and title of the random block
//and the name of the group it's from
System.out.println ( "Inserted the Autotext '" + aBlockTitles[nRandom]
+ "', shortcut '" + aBlockNames[nRandom] + "' from group '"
+ xGroupNamed.getName() );
}
}
// Go to the end of the document
mxDocCursor.gotoEnd( false );
// Insert new paragraph
mxDocText.insertControlCharacter (
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false );
// Position cursor in new paragraph
xParaCursor.gotoPreviousParagraph ( false );
// Insert a string in the new paragraph
mxDocText.insertString ( mxDocCursor, "Some text for a new autotext block", false );
// Go to the end of the document
mxDocCursor.gotoEnd( false );
}
catch (Exception e)
{
e.printStackTrace ( System.out );
}
}
Accessing Existing Tables
To access the tables contained in a text document, the text document model supports the interface com.sun.star.text.XTextTablesSupplier with one single method getTextTables()
. It returns a com.sun.star.text.TextTables service, which is a named and indexed collection, that is, tables are retrieved using com.sun.star.container.XNameAccess or com.sun.star.container.XIndexAccess.
The following snippet iterates over the text tables in a given text document object mxDoc
and colors them green.
import com.sun.star.text.XTextTablesSupplier;
import com.sun.star.container.XNameAccess;
import com.sun.star.container.XIndexAccess;
import com.sun.star.beans.XPropertySet;
...
// first query the XTextTablesSupplier interface from our document
XTextTablesSupplier xTablesSupplier = (XTextTablesSupplier)UnoRuntime.queryInterface(
XTextTablesSupplier.class, mxDoc );
// get the tables collection
XNameAccess xNamedTables = xTablesSupplier.getTextTables();
// now query the XIndexAccess from the tables collection
XIndexAccess xIndexedTables = (XIndexAccess)UnoRuntime.queryInterface(
XIndexAccess.class, xNamedTables);
// we need properties
XPropertySet xTableProps = null;
// get the tables
for (int i = 0; i < xIndexedTables.getCount(); i++) {
Object table = xIndexedTables.getByIndex(i);
// the properties, please!
xTableProps = (XPropertySet)UnoRuntime.queryInterface(
XPropertySet.class, table);
// color the table light green in format 0xRRGGBB
xTableProps.setPropertyValue("BackColor", Integer.valueOf(0xC8FFB9));
}
Text Fields
Text fields are text contents that add a second level of information to text ranges. Usually their appearance fuses together with the surrounding text, but actually the presented text comes from elsewhere. Field commands can insert the current date, page number, total page numbers, a cross-reference to another area of text, the content of certain database fields, and many variables, such as fields with changing values, into the document. There are some fields that contain their own data, where others get the data from an attached field master.
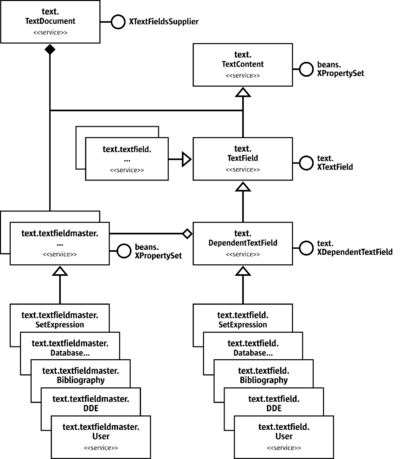
Fields are created using the com.sun.star.lang.XMultiServiceFactory of the model before inserting them using insertTextContent()
. The following text field services are available:
Text Field Service Name | Description |
---|---|
com.sun.star.text.textfield.Annotation | Annotation created through Insert - Note. |
com.sun.star.text.textfield.Author | Shows the author of the document. |
com.sun.star.text.textfield.Bibliography | Bibliographic entry created by Insert - Indexes and Tables - Bibliography Entry. The content is the source of the creation of bibliographic indexes. The sequence <PropertyValue> in the property "Fields" contains pairs of the name of the field and its content, such as:
Identifier=ABC99 BibliographicType=1 The names of the fields are defined in com.sun.star.text.BibliographyDataField. A bibliographic entry depends on com.sun.star.text.fieldmaster.Bibliography |
com.sun.star.text.textfield.Chapter | Show the chapter information. |
com.sun.star.text.textfield.CharacterCount | Show the character count of the document. |
com.sun.star.text.textfield.CombinedCharacters | Combines up to six characters as one text object that is formatted in two lines. |
com.sun.star.text.textfield.ConditionalText | Inserts text depending on a condition. |
com.sun.star.text.textfield.Database | The form letter field showing the content from a database. Depends on com.sun.star.text.fieldmaster.Database. |
com.sun.star.text.textfield.DatabaseName | Shows the name of a database. |
com.sun.star.text.textfield.DatabaseNextSet | Increments the cursor that points to a database selection. |
com.sun.star.text.textfield.DatabaseNumberOfSet | Shows the set number of a database cursor. |
com.sun.star.text.textfield.DatabaseSetNumber | Databases - Any Record. Sets the number of a database cursor. |
com.sun.star.text.textfield.DateTime | Shows a date or time value. |
com.sun.star.text.textfield.DDE | Shows the result of a DDE operation. Depends on com.sun.star.text.fieldmaster.DDE. |
com.sun.star.text.textfield.docinfo.ChangeAuthor | Shows the name of the author of the last change of the document. |
com.sun.star.text.textfield.docinfo.ChangeDateTime | Shows the date and time of the last change of the document. |
com.sun.star.text.textfield.docinfo.CreateAuthor | Shows the name of the creator of the document. |
com.sun.star.text.textfield.docinfo.CreateDateTime | Shows the date and time of the document creation. |
com.sun.star.text.textfield.docinfo.Custom | Shows the content of a user defined field of the document info. |
com.sun.star.text.textfield.docinfo.Description | Shows the description contained in the document information. |
com.sun.star.text.textfield.docinfo.EditTime | Shows the time of the editing of the document. |
com.sun.star.text.textfield.docinfo.Keywords | Shows the keywords contained in the document info. |
com.sun.star.text.textfield.docinfo.PrintAuthor | Shows the name of the author of the last printing. |
com.sun.star.text.textfield.docinfo.PrintDateTime | Shows the date and time of the last printing. |
com.sun.star.text.textfield.docinfo.Revision | Shows the revision contained in the document info. |
com.sun.star.text.textfield.docinfo.Subject | Shows the subject contained in the document info. |
com.sun.star.text.textfield.docinfo.Title | Shows the title contained in the document info. |
com.sun.star.text.textfield.EmbeddedObjectCount | Shows the number of embedded objects contained in the document. |
com.sun.star.text.textfield.ExtendedUser | Shows the user data of the Office user. |
com.sun.star.text.textfield.FileName | Shows the file name (URL) of the document. |
com.sun.star.text.textfield.GetExpression | Variables - Show Variable. Shows the value set by the previous occurrence of SetExpression. |
com.sun.star.text.textfield.GetReference | References - Insert Reference. Shows a reference to a reference mark, bookmark, number range field, footnote or an endnote. |
com.sun.star.text.textfield.GraphicObjectCount | Shows the number of graphic object in the document. |
com.sun.star.text.textfield.HiddenParagraph | Depending on a condition, the field hides the paragraph it is contained in. |
com.sun.star.text.textfield.HiddenText | Depending on a condition the field shows or hides a text. |
com.sun.star.text.textfield.Input | The field activates a dialog to input a value that changes a related User field or SetExpression field.
|
com.sun.star.text.textfield.InputUser | The field activates a dialog to input a string that is displayed by the field. This field is not connected to variables. |
com.sun.star.text.textfield.JumpEdit | A placeholder field with an attached interaction to insert text, a text table, text frame, graphic object or an OLE object. |
com.sun.star.text.textfield.Macro | A field connected to a macro that is executed on a click to the field. To execute such a macro, use the dispatch (cf. Appendix). |
com.sun.star.text.textfield.PageCount | Shows the number of pages of the document. |
com.sun.star.text.textfield.PageNumber | Shows the page number (current, previous, next). |
com.sun.star.text.textfield.ParagraphCount | Shows the number of paragraphs contained in the document. |
com.sun.star.text.textfield.ReferencePageGet | Displays the page number with respect to the reference point, that is determined by the text field ReferencePageSet. |
com.sun.star.text.textfield.ReferencePageSet | Inserts a starting point for additional page numbers that can be switched on or off. |
com.sun.star.text.textfield.Script | Contains a script or a URL to a script. |
com.sun.star.text.textfield.SetExpression | Variables - Set Variable. A variable field. The value is valid until the next occurrence of SetExpression field. The actual value depends on com.sun.star.text.fieldmaster.SetExpression.
|
com.sun.star.text.textfield.TableCount | Shows the number of text tables of the document. |
com.sun.star.text.textfield.TableFormula | Contains a formula to calculate in a text table. |
com.sun.star.text.textfield.TemplateName | Shows the name of the template the current document is created from. |
com.sun.star.text.textfield.User | Variables - User Field. Creates a global document variable and displays it whenever this field occurs in the text. Depends on com.sun.star.text.fieldmaster.User. |
com.sun.star.text.textfield.WordCount | Shows the number of words contained in the document. |
All fields support the interfaces com.sun.star.text.XTextField, com.sun.star.util.XUpdatable, com.sun.star.text.XDependentTextField and the service com.sun.star.text.TextContent.
The method getPresentation()
of the interface com.sun.star.text.XTextField returns the textual representation of the result of the text field operation, such as a date, time, variable value, or the command, such as CHAPTER, TIME (fixed) depending on the boolean parameter.
The method update()
of the interface com.sun.star.util.XUpdatable affects only the following field types:
- Date and time fields are set to the current date and time.
- The
ExtendedUser
fields that show parts of the user data set for LibreOffice, such as the Name, City, Phone No. and the Author fields that are set to the current values. - The
FileName
fields are updated with the current name of the file. - The
DocInfo.XXX
fields are updated with the current document info of the document.
All other fields ignore calls to update()
.
Some of these fields need a field master that provides the data that appears in the field. This applies to the field types Database
, SetExpression
, DDE
, User
and Bibliography
. The interface com.sun.star.text.XDependentTextField handles these pairs of FieldMasters and TextFields. The method attachTextFieldMaster()
must be called prior to inserting the field into the document. The method getTextFieldMaster()
does not work unless the dependent field is inserted into the document.
To create a valid text field master, the instance has to be created using the com.sun.star.lang.XMultiServiceFactory interface of the model with the appropriate service name:
Text Field Master Service Names | Description |
---|---|
com.sun.star.text.fieldmaster.User | Contains the global variable that is created and displayed by the fieldtype com.sun.star.text.textfield.User. |
com.sun.star.text.fieldmaster.DDE | The DDE command for a com.sun.star.text.textfield.DDE. |
com.sun.star.text.fieldmaster.SetExpression | Numbering settings if the corresponding com.sun.star.text.textfield.SetExpression is a number range. A sub type of expression. |
com.sun.star.text.fieldmaster.Database | Data source definition for a com.sun.star.text.textfield.Database. |
com.sun.star.text.fieldmaster.Bibliography | Display settings and sorting for com.sun.star.text.textfield.Bibliography. |
The property Name has to be set after the field instance is created, except for the Database
field master type where the properties DatabaseName
, DatabaseTableName
, DataColumnName
and DatabaseCommandType
are set instead of the Name
property.
To access existing text fields and field masters, use the interface com.sun.star.text.XTextFieldsSupplier that is implemented at the text document model.
Its method getTextFields()
returns a com.sun.star.text.TextFields container which is a com.sun.star.container.XEnumerationAccess and can be refreshed through the refresh()
method in its interface com.sun.star.util.XRefreshable.
Its method getTextFieldMasters()
returns a com.sun.star.text.TextFieldMasters container holding the text field masters of the document. This container provides a com.sun.star.container.XNameAccess interface. All field masters, except for Database
are named by the service name followed by the name of the field master. The Database
field masters create their names by appending the DatabaseName
, DataTableName
and DataColumnName
to the service name.
Consider the following examples for this naming convention:
"com.sun.star.text.fieldmaster.SetExpression.Illustration" | Master for Illustration number range. Number ranges are built-in SetExpression fields present in every document.
|
"com.sun.star.text.fieldmaster.User.Company" | Master for User field (global document variable), inserted with display name Company.
|
"com.sun.star.text.fieldmaster.Database.Bibliography.biblio.Identifier" | Master for form letter field referring to the column Identifier in the built-in dbase database table biblio.
|
Each text field master has a property InstanceName
that contains its name in the format of the related container.
Some SetExpression
text field masters are always available if they are not deleted. These are the masters with the names Text, Illustration, Table and Drawing. They are predefined as number range field masters used for captions of text frames, graphics, text tables and drawings. Note that these predefined names are internal names that are usually not used at the user interface.
The following methods show how to create and insert text fields.
/** This method inserts both a date field and a user field containing the number '42'
*/
protected void TextFieldExample() {
try {
// Use the text document's factory to create a DateTime text field,
// and access it's
// XTextField interface
XTextField xDateField = UnoRuntime.queryInterface(
XTextField.class, mxDocFactory.createInstance(
"com.sun.star.text.textfield.DateTime"));
// Insert it at the end of the document
mxDocText.insertTextContent ( mxDocText.getEnd(), xDateField, false );
// Use the text document's factory to create a user text field,
// and access it's XDependentTextField interface
XDependentTextField xUserField = UnoRuntime.queryInterface (
XDependentTextField.class, mxDocFactory.createInstance(
"com.sun.star.text.textfield.User"));
// Create a fieldmaster for our newly created User Text field, and access it's
// XPropertySet interface
XPropertySet xMasterPropSet = UnoRuntime.queryInterface(
XPropertySet.class, mxDocFactory.createInstance(
"com.sun.star.text.fieldmaster.User"));
// Set the name and value of the FieldMaster
xMasterPropSet.setPropertyValue ("Name", "UserEmperor");
xMasterPropSet.setPropertyValue ("Value", Integer.valueOf(42));
// Attach the field master to the user field
xUserField.attachTextFieldMaster (xMasterPropSet);
// Move the cursor to the end of the document
mxDocCursor.gotoEnd(false);
// insert a paragraph break using the XSimpleText interface
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
// Insert the user field at the end of the document
mxDocText.insertTextContent(mxDocText.getEnd(), xUserField, false);
} catch (Exception e) {
e.printStackTrace (System.out);
}
}
Bookmarks
A Bookmark is a text content that marks a position inside of a paragraph or a text selection that supports the com.sun.star.text.TextContent service. To search for a bookmark, the text document model implements the interface com.sun.star.text.XBookmarksSupplier that supplies a collection of the bookmarks. The collection supports the service com.sun.star.text.Bookmarks which consists of com.sun.star.container.XNameAccess and com.sun.star.container.XIndexAccess.
The bookmark name can be read and changed through its (com.sun.star.container.XNamed) interface.
To insert, remove or change text, or attributes starting from the position of a bookmark, retrieve its com.sun.star.text.XTextRange by calling getAnchor()
at its com.sun.star.text.XTextContent interface. Then use getString()
or setString()
at the XTextRange
, or pass this XTextRange
to methods expecting a text range, such as createTextCursorByRange(), insertString() or insertTextContent().
Use the createInstance
method of the com.sun.star.lang.XMultiServiceFactory interface provided by the text document model to insert an new bookmark into the document. The service name is "com.sun.star.text.Bookmark
". Then use the bookmark's com.sun.star.container.XNamed interface and call setName()
. If no name is set, LibreOffice makes up generic names, such as Bookmark1 and Bookmark2. Similarly, if a name is used that is not unique, writer automatically appends a number to the bookmark name. The bookmark object obtained from createInstance()
can only be inserted once.
// inserting and retrieving a bookmark
Object bookmark = mxDocFactory.createInstance ( "com.sun.star.text.Bookmark" );
// name the bookmark
XNamed xNamed = (XNamed)UnoRuntime.queryInterface (
XNamed.class, bookmark );
xNamed.setName("MyUniqueBookmarkName");
// get XTextContent interface
XTextContent xTextContent = (XTextContent)UnoRuntime.queryInterface (
XTextContent.class, bookmark );
// insert bookmark at the end of the document
// instead of mxDocText.getEnd you could use a text cursor's XTextRange interface or any XTextRange
mxDocText.insertTextContent ( mxDocText.getEnd(), xTextContent, false );
// query XBookmarksSupplier from document model and get bookmarks collection
XBookmarksSupplier xBookmarksSupplier = (XBookmarksSupplier)UnoRuntime.queryInterface(
XBookmarksSupplier.class, xWriterComponent);
XNameAccess xNamedBookmarks = xBookmarksSupplier.getBookmarks();
// retrieve bookmark by name
Object foundBookmark = xNamedBookmarks.getByName("MyUniqueBookmarkName");
XTextContent xFoundBookmark = (XTextContent)UnoRuntime.queryInterface(
XTextContent.class, foundBookmark);
// work with bookmark
XTextRange xFound = xFoundBookmark.getAnchor();
xFound.setString(" The throat mike, glued to her neck, "
+ "looked as much as possible like an analgesic dermadisk.");
Indexes and Index Marks
Indexes are text contents that pull together information that is dispersed over the document. They can contain chapter headings, locations of key words, locations of arbitrary index marks and locations of text objects, such as illustrations, objects or tables. In addition, LibreOffice features a bibliographical index.
Indexes
The following index services are available in LibreOffice:
Index Service Name | Description |
---|---|
com.sun.star.text.DocumentIndex | alphabetical index |
com.sun.star.text.ContentIndex | table of contents |
com.sun.star.text.UserIndex | user defined index |
com.sun.star.text.IllustrationIndex | table of all illustrations contained in the document |
com.sun.star.text.ObjectIndex | table of all objects contained in the document |
com.sun.star.text.TableIndex | table of all text tables contained in the document |
com.sun.star.text.Bibliography | bibliographical index |
To access the indexes of a document, the text document model supports the interface com.sun.star.text.XDocumentIndexesSupplier with a single method getDocumentIndexes()
. The returned object is a com.sun.star.text.DocumentIndexes service supporting the interfaces com.sun.star.container.XIndexAccess and com.sun.star.container.XNameAccess.
All indexes support the services com.sun.star.text.TextContent and com.sun.star.text.BaseIndex that include the interface com.sun.star.text.XDocumentIndex. This interface is used to access the service name of the index and update the current content of an index:
string getServiceName() void update()
Furthermore, indexes have properties and a name, and support:
- provides the properties that determine how the index is created and which elements are included into the index.
- provides a unique name of the index, not necessarily the title of the index.
An index is usually composed of two text sections which are provided as properties. The provided property ContentSection
includes the complete index and the property HeaderSection
contains the title if there is one. They enable the index to have background or column attributes independent of the surrounding page format valid at the index position. In addition, there may be different settings for the content and the heading of the index. However, these text sections are not part of the document's text section container.
The indexes are structured by levels. The number of levels depends on the index type. The content index has ten levels, corresponding to the number of available chapter numbering levels, which is ten. Alphabetical indexes have four levels, one of which is used to insert separators, that are usually characters that show the alphabet. The bibliography has 22 levels, according to the number of available bibliographical type entries (com.sun.star.text.BibliographyDataType). All other index types only have one level.
For all levels, define a separate structure that is provided by the property LevelFormat
of the service com.sun.star.text.BaseIndex. LevelFormat
contains the various levels as a com.sun.star.container.XIndexReplace object. Each level is a sequence of com.sun.star.beans.PropertyValues which are defined in the service com.sun.star.text.DocumentIndexLevelFormat. Although LevelFormat
provides a level for the heading, changing that level is not supported.
Each com.sun.star.beans.PropertyValues sequence has to contain at least one com.sun.star.beans.PropertyValue with the name TokenType
. This PropertyValue
struct must contain one of the following string values in its Value member variable:
TokenType Value (String) | Meaning | Additional Sequence Members (optional) |
---|---|---|
"TokenEntryNumber "
|
The number of an entry. This is only supported in tables of content and it marks the appearance of the chapter number. | CharacterStyleName
|
"TokenEntryText "
|
Text of the entry, for example, it might contain the heading text in tables of content or the name of a text reference in a bibliography. | CharacterStyleName
|
"TokenTabStop "
|
Marks a tab stop to be inserted. | TabStopPosition
|
"TokenText "
|
Inserted text. | CharacterStyleName
|
"TokenPageNumber "
|
Marks the insertion of the page number. | CharacterStyleName
|
"TokenChapterInfo "
|
Marks the insertion of a chapter field to be inserted. Only supported in alphabetical indexes. | CharacterStyleName
ChapterFormat |
"TokenHyperlinkStart "
|
Start of a hyperlink to jump to the referred heading. Only supported in tables of content. | |
"TokenHyperlinkEnd "
|
End of a hyperlink to jump to the referred heading. Only supported in tables of content. | |
"TokenBibliographyDataField "
|
Identifies one of the 30 possible BibliographyDataFields. The number 30 comes from the IDL reference of BilbliographyDataFields. | BibliographyDataField
|
An example for such a sequence of PropertyValue
struct could be constructed like this:
PropertyValue[] indexTokens = new PropertyValue[1]; indexTokens [0] = new PropertyValue(); indexTokens [0].Name = "TokenType"; indexTokens [0].Value = "TokenHyperlinkStart";
The following table explains the sequence members which can be present, in addition to the TokenType
member, as mentioned above.
Additional Properties of com.sun.star.text.DocumentIndexLevelFormat | |
---|---|
CharacterStyleName
|
string - Name of the character style that has to be applied to the appearance of the entry.
|
TabStopPosition
|
long - Position of the tab stop in 1/100 mm.
|
TabStopRightAligned
|
boolean - The tab stop is to be inserted at the end of the line and right aligned. This is used before page number entries.
|
TabStopFillCharacter
|
string - The first character of this string is used as a fill character for the tab stop.
|
ChapterFormat
|
short - Type of the chapter info as defined in com.sun.star.text.ChapterFormat.
|
BibliographyDataField
|
Type of the bibliographical entry as defined in com.sun.star.text.BibliographyDataField. |
Index marks
Index marks are text contents whose contents and positions are collected and displayed in indexes.
To access all index marks that are related to an index, use the property IndexMarks
of the index. It contains a sequence of com.sun.star.text.XDocumentIndexMark interfaces.
All index marks support the service com.sun.star.text.BaseIndexMark that includes com.sun.star.text.TextContent. Also, they all implement the interfaces com.sun.star.text.XDocumentIndexMark and com.sun.star.beans.XPropertySet.
The XDocumentIndexMark
inherits from XTextContent
and defines two methods:
string getMarkEntry() void setMarkEntry( [in] string anIndexEntry)
LibreOffice supports three different index mark services:
- com.sun.star.text.DocumentIndexMark for entries in alphabetical indexes.
- com.sun.star.text.UserIndexMark for user defined indexes.
- com.sun.star.text.ContentIndexMark for entries in tables of content which are independent from chapter headings.
An index mark can be set at a point in text or it can mark a portion of a paragraph, usually a word. It cannot contain text across paragraph breaks. If the index mark does not include text, the BaseIndexMark
property AlternativeText
has to be set, otherwise there will be no string to insert into the index.
Inserting ContentIndexMarks
and a table of contents index:
/** This method demonstrates how to insert indexes and index marks
*/
protected void IndexExample ()
{
try
{
// Go to the end of the document
mxDocCursor.gotoEnd( false );
// Insert a new paragraph and position the cursor in it
mxDocText.insertControlCharacter ( mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false );
XParagraphCursor xParaCursor = (XParagraphCursor)
UnoRuntime.queryInterface( XParagraphCursor.class, mxDocCursor );
xParaCursor.gotoPreviousParagraph ( false );
// Create a new ContentIndexMark and get it's XPropertySet interface
XPropertySet xEntry = UnoRuntime.queryInterface( XPropertySet.class,
mxDocFactory.createInstance ( "com.sun.star.text.ContentIndexMark" ) );
// Set the text to be displayed in the index
xEntry.setPropertyValue ( "AlternativeText", "Big dogs! Falling on my head!" );
// The Level property _must_ be set
xEntry.setPropertyValue ( "Level", new Short ( (short) 1 ) );
// Create a ContentIndex and access it's XPropertySet interface
XPropertySet xIndex = UnoRuntime.queryInterface( XPropertySet.class,
mxDocFactory.createInstance ( "com.sun.star.text.ContentIndex" ) );
// Again, the Level property _must_ be set
xIndex.setPropertyValue ( "Level", new Short ( (short) 10 ) );
// Access the XTextContent interfaces of both the Index and the IndexMark
XTextContent xIndexContent = UnoRuntime.queryInterface(
XTextContent.class, xIndex );
XTextContent xEntryContent = UnoRuntime.queryInterface(
XTextContent.class, xEntry );
// Insert both in the document
mxDocText.insertTextContent ( mxDocCursor, xEntryContent, false );
mxDocText.insertTextContent ( mxDocCursor, xIndexContent, false );
// Get the XDocumentIndex interface of the Index
XDocumentIndex xDocIndex = UnoRuntime.queryInterface(
XDocumentIndex.class, xIndex );
// And call it's update method
xDocIndex.update();
}
catch (Exception e)
{
e.printStackTrace ( System.out );
}
}
Reference Marks
A reference mark is a text content that is used as a target for com.sun.star.text.textfield.GetReference text fields. These text fields show the contents of reference marks in a text document and allows the user to jump to the reference mark. Reference marks support the com.sun.star.text.XTextContent and com.sun.star.container.XNamed interfaces. They can be accessed by using the text document's com.sun.star.text.XReferenceMarksSupplier interface that defines a single method getReferenceMarks()
.
The returned collection is a com.sun.star.text.ReferenceMarks service which has a com.sun.star.container.XNameAccess and a com.sun.star.container.XIndexAccess interface.
/** This method demonstrates how to create and insert reference marks, and GetReference Text Fields
*/
protected void ReferenceExample () {
try {
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Insert a paragraph break
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
// Get the Paragraph cursor
XParagraphCursor xParaCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, mxDocCursor);
// Move the cursor into the new paragraph
xParaCursor.gotoPreviousParagraph(false);
// Create a new ReferenceMark and get it's XNamed interface
XNamed xRefMark = UnoRuntime.queryInterface(XNamed.class,
mxDocFactory.createInstance("com.sun.star.text.ReferenceMark"));
// Set the name to TableHeader
xRefMark.setName("TableHeader");
// Get the TextTablesSupplier interface of the document
XTextTablesSupplier xTableSupplier = UnoRuntime.queryInterface(
XTextTablesSupplier.class, mxDoc);
// Get an XIndexAccess of TextTables
XIndexAccess xTables = UnoRuntime.queryInterface(
XIndexAccess.class, xTableSupplier.getTextTables());
// We've only inserted one table, so get the first one from index zero
XTextTable xTable = UnoRuntime.queryInterface(
XTextTable.class, xTables.getByIndex(0));
// Get the first cell from the table
XText xTableText = UnoRuntime.queryInterface(
XText.class, xTable.getCellByName("A1"));
// Get a text cursor for the first cell
XTextCursor xTableCursor = xTableText.createTextCursor();
// Get the XTextContent interface of the reference mark so we can insert it
XTextContent xContent = UnoRuntime.queryInterface(
XTextContent.class, xRefMark);
// Insert the reference mark into the first cell of the table
xTableText.insertTextContent (xTableCursor, xContent, false);
// Create a 'GetReference' text field to refer to the reference mark we just inserted,
// and get it's XPropertySet interface
XPropertySet xFieldProps = UnoRuntime.queryInterface(
XPropertySet.class, mxDocFactory.createInstance(
"com.sun.star.text.textfield.GetReference"));
// Get the XReferenceMarksSupplier interface of the document
XReferenceMarksSupplier xRefSupplier = UnoRuntime.queryInterface(
XReferenceMarksSupplier.class, mxDoc);
// Get an XNameAccess which refers to all inserted reference marks
XNameAccess xMarks = UnoRuntime.queryInterface(XNameAccess.class,
xRefSupplier.getReferenceMarks());
// Put the names of each reference mark into an array of strings
String[] aNames = xMarks.getElementNames();
// Make sure that at least 1 reference mark actually exists
// (well, we just inserted one!)
if (aNames.length > 0) {
// Output the name of the first reference mark ('TableHeader')
System.out.println ("GetReference text field inserted for ReferenceMark : "
+ aNames[0]);
// Set the SourceName of the GetReference text field to 'TableHeader'
xFieldProps.setPropertyValue("SourceName", aNames[0]);
// specify that the source is a reference mark (could also be a footnote,
// bookmark or sequence field)
xFieldProps.setPropertyValue ("ReferenceFieldSource", new Short(
ReferenceFieldSource.REFERENCE_MARK));
// We want the reference displayed as 'above' or 'below'
xFieldProps.setPropertyValue("ReferenceFieldPart",
new Short (ReferenceFieldPart.UP_DOWN));
// Get the XTextContent interface of the GetReference text field
XTextContent xRefContent = UnoRuntime.queryInterface(
XTextContent.class, xFieldProps);
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Make some text to precede the reference
mxDocText.insertString(mxDocText.getEnd(), "The table ", false);
// Insert the text field
mxDocText.insertTextContent(mxDocText.getEnd(), xRefContent, false);
// And some text after the reference..
mxDocText.insertString( mxDocText.getEnd(),
" contains the sum of some random numbers.", false);
// Refresh the document
XRefreshable xRefresh = UnoRuntime.queryInterface(
XRefreshable.class, mxDoc);
xRefresh.refresh();
}
} catch (Exception e) {
e.printStackTrace(System.err);
}
}
The name of a reference mark can be used in a com.sun.star.text.textfield.GetReference text field to refer to the position of the reference mark.
Footnotes and Endnotes
Footnotes and endnotes are text contents that provide background information for the reader that appears in page footers or at the end of a document.
Footnotes and endnotes implement the service com.sun.star.text.Footnote that includes com.sun.star.text.TextContent. The Footnote
service has the interfaces com.sun.star.text.XText and com.sun.star.text.XFootnote that inherit from com.sun.star.text.XTextContent. The XFootnote
introduces the following methods:
string getLabel() void setLabel( [in] string aLabel)
The Footnote
service defines a property ReferenceId
that is used for import and export, and contains an internal sequential number.
The interface com.sun.star.text.XText which is provided by the com.sun.star.text.Footnote service accesses the text object in the footnote area where the footnote text is located. It is not allowed to insert text tables into this text object.
While footnotes can be placed at the end of a page or the end of a document, endnotes always appear at the end of a document. Endnote numbering is separate from footnote numbering. Footnotes are accessed using the com.sun.star.text.XFootnotesSupplier interface of the text document through the method getFootNotes()
. Endnotes are accessed similarly by calling getEndnotes()
at the text document's com.sun.star.text.XEndnotesSupplier interface. Both of these methods return a com.sun.star.container.XIndexAccess.
A label is set for a footnote or endnote to determine if automatic footnote numbering is used. If no label is set (= empty string), the footnote is labeled automatically. There are footnote and endnote settings that specify how the automatic labeling is formatted. These settings are obtained from the document model using the interfaces com.sun.star.text.XFootnotesSupplier and com.sun.star.text.XEndnotesSupplier. The corresponding methods are getFootnoteSettings()
and getEndnoteSettings()
. The object received is a com.sun.star.beans.XPropertySet and has the properties described in com.sun.star.text.FootnoteSettings:
Properties of com.sun.star.text.FootnoteSettings | |
---|---|
AnchorCharStyleName | string - Contains the name of the character style that is used for the label in the document text.
|
CharStyleName | string - Contains the name of the character style that is used for the label in front of the footnote/endnote text.
|
NumberingType | short - Contains the numbering type for the numbering of the footnotes or endnotes.
|
PageStyleName | string - Contains the page style that is used for the page that contains the footnote or endnote texts.
|
ParaStyleName | string - Contains the paragraph style that is used for the footnote or endnote text.
|
Prefix | string - Contains the prefix for the footnote or endnote symbol.
|
StartAt | short - Contains the first number of the automatic numbering of footnotes or endnotes.
|
Suffix | string - Contains the suffix for the footnote/endnote symbol.
|
BeginNotice | [optional] string - Contains the string at the restart of the footnote text after a break.
|
EndNotice | [optional] string - Contains the string at the end of a footnote part in front of a break.
|
FootnoteCounting | [optional] boolean - Contains the type of the counting for the footnote numbers
|
PositionEndOfDoc | [optional] boolean - If true, the footnote text is shown at the end of the document.
|
The Footnotes service applies to footnotes and endnotes.
The following sample works with footnotes
/** This method demonstrates how to create and insert footnotes, and how to access the
XFootnotesSupplier interface of the document
*/
protected void FootnoteExample ()
{
try
{
// Create a new footnote from the document factory and get it's
// XFootnote interface
XFootnote xFootnote = UnoRuntime.queryInterface( XFootnote.class,
mxDocFactory.createInstance ( "com.sun.star.text.Footnote" ) );
// Set the label to 'Numbers'
xFootnote.setLabel ( "Numbers" );
// Get the footnotes XTextContent interface so we can...
XTextContent xContent = UnoRuntime.queryInterface (
XTextContent.class, xFootnote );
// ...insert it into the document
mxDocText.insertTextContent ( mxDocCursor, xContent, false );
// Get the XFootnotesSupplier interface of the document
XFootnotesSupplier xFootnoteSupplier = UnoRuntime.queryInterface(
XFootnotesSupplier.class, mxDoc );
// Get an XIndexAccess interface to all footnotes
XIndexAccess xFootnotes = UnoRuntime.queryInterface (
XIndexAccess.class, xFootnoteSupplier.getFootnotes() );
// Get the XFootnote interface to the first footnote inserted ('Numbers')
XFootnote xNumbers = UnoRuntime.queryInterface (
XFootnote.class, xFootnotes.getByIndex( 0 ) );
// Get the XSimpleText interface to the Footnote
XSimpleText xSimple = UnoRuntime.queryInterface (
XSimpleText.class, xNumbers );
// Create a text cursor for the foot note text
XTextRange xRange = UnoRuntime.queryInterface (
XTextRange.class, xSimple.createTextCursor() );
// And insert the actual text of the footnote.
xSimple.insertString (
xRange, " The numbers were generated by using java.util.Random", false );
}
catch (Exception e)
{
e.printStackTrace ( System.out );
}
}
Shape Objects in Text
Base Frames vs. Drawing Shapes
Shape objects are text contents that act independently of the ordinary text flow. The surrounding text may wrap around them. Shape objects can lie in front or behind text, and be anchored to paragraphs or characters in the text. Anchoring allows the shape objects to follow the paragraphs and characters while the user is writing. Currently, there are two different kinds of shape objects in LibreOffice, base frames and drawing shapes.
Base Frames
The first group are shape objects that are com.sun.star.text.BaseFrames. The three services com.sun.star.text.TextFrame, com.sun.star.text.TextGraphicObject and com.sun.star.text.TextEmbeddedObject are all based on the service com.sun.star.text.BaseFrame. The TextFrames
contain an independent text area that can be positioned freely over ordinary text. The TextGraphicObjects
are bitmaps or vector oriented images in a format supported by LibreOffice internally. The TextEmbeddedObjects
are areas containing a document type other than the document they are embedded in, such as charts, formulas, internal LibreOffice documents (Calc/Draw/Impress), or OLE objects.
The TextFrames
, TextGraphicObjects
and TextEmbeddedObjects
in a text are supplied by their corresponding supplier interfaces at the document model:
- com.sun.star.text.XTextFramesSupplier
- com.sun.star.text.XTextGraphicObjectsSupplier
- com.sun.star.text.XTextEmbeddedObjectsSupplier.
These interfaces all have one single get method that supplies the respective Shape objects collection:
com::sun::star::container::XNameAccess getTextFrames() com::sun::star::container::XNameAccess getTextEmbeddedObjects() com::sun::star::container::XNameAccess getTextGraphicObjects()
The method getTextFrames()
returns a com.sun.star.text.TextFrames collection, getTextEmbeddedObjects()
returns a com.sun.star.text.TextEmbeddedObjects collection and getTextGraphicObjects()
yields a com.sun.star.text.TextGraphicObjects collection. All of these collections support com.sun.star.container.XIndexAccess and com.sun.star.container.XNameAccess. The TextFrames
collection may (optional) support the com.sun.star.container.XContainer interface to broadcast an event when an Element
is added to the collection. However, the current implementation of the TextFrames
collection does not support this.
The service com.sun.star.text.BaseFrame defines the common properties and interfaces of text frames, graphic objects and embedded objects. It includes the services com.sun.star.text.BaseFrameProperties and com.sun.star.text.TextContent, and defines the following interfaces.
The position and size of a BaseFrame
is covered by com.sun.star.drawing.XShape. All BaseFrame
objects share a majority of the core implementation of drawing objects. Therefore, they have a position and size on the DrawPage
.
The name of a BaseFrame
is set and read through com.sun.star.container.XNamed. The names of the frame objects have to be unique for text frames, graphic objects and embedded objects, respectively.
The com.sun.star.beans.XPropertySet has to be present, because many aspects of BaseFrames
are controlled through properties.
The interface com.sun.star.document.XEventsSupplier is not a part of the BaseFrame
service, but is available in text frames, graphic objects and embedded objects. This interface provides access to the event macros that may be attached to the object in the GUI.
The properties of BaseFrames
are those of the service com.sun.star.text.TextContent, as well there is a number of frame properties defined in the service com.sun.star.text.BaseFrameProperties:
Properties of com.sun.star.text.BaseFrameProperties | |
---|---|
AnchorPageNo | short - Contains the number of the page where the objects are anchored.
|
AnchorFrame | com.sun.star.text.XTextFrame. Contains the text frame the current frame is anchored to. |
BackColor | long - Contains the color of the background of the object.
|
BackGraphicURL | string - Contains the URL for the background graphic.
|
BackGraphicFilter | string - Contains the name of the file filter for the background graphic.
|
BackGraphicLocation | Determines the position of the background graphic according to com.sun.star.style.GraphicLocation. |
LeftBorder | struct com.sun.star.table.BorderLine. Contains the left border of the object.
|
RightBorder | struct com.sun.star.table.BorderLine. Contains the right border of the object.
|
TopBorder | struct com.sun.star.table.BorderLine. Contains the top border of the object.
|
BottomBorder | struct com.sun.star.table.BorderLine. Contains the bottom border of the object.
|
BorderDistance | long - Contains the distance from the border to the object.
|
LeftBorderDistance | long - Contains the distance from the left border to the object.
|
RightBorderDistance | long - Contains the distance from the right border to the object.
|
TopBorderDistance | long - Contains the distance from the top border to the object.
|
BottomBorderDistance | long - Contains the distance from the bottom border to the object.
|
BackTransparent | boolean - If true, the property BackColor is ignored.
|
ContentProtected | boolean - Determines if the content is protected.
|
LeftMargin | long - Contains the left margin of the object.
|
RightMargin | long - Contains the right margin of the object.
|
TopMargin | long - Contains the top margin of the object.
|
BottomMargin | long - Contains the bottom margin of the object.
|
Height | long - Contains the height of the object (1/100 mm).
|
Width | long - Contains the width of the object (1/100 mm).
|
RelativeHeight | short - Contains the relative height of the object.
|
RelativeWidth | short - Contains the relative width of the object.
|
IsSyncWidthToHeight | boolean - Determines if the width follows the height.
|
IsSyncHeightToWidth | boolean - Determines if the height follows the width.
|
HoriOrient | short - Determines the horizontal orientation of the object according to com.sun.star.text.HoriOrientation.
|
HoriOrientPosition | long - Contains the horizontal position of the object (1/100 mm).
|
HoriOrientRelation | short - Determines the environment of the object the orientation is related according to com.sun.star.text.RelOrientation.
|
VertOrient | short - Determines the vertical orientation of the object according to com.sun.star.text.VertOrientation.
|
VertOrientPosition | long - Contains the vertical position of the object (1/100 mm). Valid only if TextEmbeddedObject::VertOrient is VertOrientation::NONE .
|
VertOrientRelation | short - Determines the environment of the object the orientation is related according to com.sun.star.text.RelOrientation.
|
HyperLinkURL | string - Contains the URL of a hyperlink that is set at the object.
|
HyperLinkTarget | string - Contains the name of the target for a hyperlink that is set at the object.
|
HyperLinkName | string - Contains the name of the hyperlink that is set at the object.
|
Opaque | boolean - Determines if the object is opaque or transparent for text.
|
PageToggle | boolean - Determines if the object is mirrored on even pages.
|
PositionProtected | boolean - Determines if the position is protected.
|
boolean - Determines if the object is included in printing.
| |
ShadowFormat | struct com.sun.star.table.ShadowFormat. Contains the type of the shadow of the object.
|
ServerMap | boolean - Determines if the object gets an image map from a server.
|
Size | struct com.sun.star.awt.Size. Contains the size of the object.
|
SizeProtected | boolean - Determines if the size is protected.
|
Surround | [deprecated]. Determines the type of the surrounding text. |
SurroundAnchorOnly | boolean - Determines if the text of the paragraph where the object is anchored, wraps around the object.
|
Drawing Shapes
The second group of shape objects are the varied drawing shapes that can be inserted into text, such as rectangles and ellipses. They are based on com.sun.star.text.Shape. The service text.Shape
includes Shape, but adds a number of properties related to shapes in text (cf. Drawing Shapes below). In addition, drawing shapes support the interface com.sun.star.text.XTextContent so that they can be inserted into an XText.
There are no specialized supplier interfaces for drawing shapes. All the drawing shapes on the DrawPage
object are supplied by the document model's com.sun.star.drawing.XDrawPageSupplier and its single method:
com::sun::star::drawing::XDrawPage getDrawPage()
Text Frames
A text frame is a com.sun.star.text.TextFrame service consisting of com.sun.star.text.BaseFrame and the interface com.sun.star.text.XTextFrame.The XTextFrame
is based on com.sun.star.text.XTextContent and introduces one method to provide the XText
of the frame:
com::sun::star::text::XText getText()
The properties of com.sun.star.text.TextFrame that add to the BaseFrame
are the following:
Properties of com.sun.star.text.TextFrame | |
---|---|
FrameHeightAbsolute | long - Contains the metric height value of the frame.
|
FrameWidthAbsolute | long - Contains the metric width value of the frame.
|
FrameWidthPercent | byte - Specifies a width relative to the width of the surrounding text.
|
FrameHeightPercent | byte - Specifies a width relative to the width of the surrounding text.
|
FrameIsAutomaticHeight | boolean - If "AutomaticHeight" is set, the object grows if it is required by the frame content.
|
SizeType | short - Determines the interpretation of the height and relative height properties.
|
Additionally, text frames are com.sun.star.text.Text services and support all of its interfaces, except for com.sun.star.text.XTextRangeMover.
Text frames can be connected to a chain, that is, the text of the first text frame flows into the next chain element if it does not fit. The properties ChainPrevName
and ChainNextName
are provided to take advantage of this feature. They contain the names of the predecessor and successor of a frame. All frames have to be empty to chain frames, except for the first member of the chain.
Chained Text Frame Property | |
---|---|
ChainPrevName | string - Name of the predecessor of the frame.
|
ChainNextName | string - Name of the successor of the frame.
|
The effect at the API is that the visible text content of the chain members is only accessible at the first frame in the chain. The content of the following chain members is not shown when chained before their content is set.
The API reference does not know the properties above. Instead, it specifies a com.sun.star.text.ChainedTextFrame with an XChainable
interface, but this is not yet supported by text frames.
The following example uses text frames:
/** This method shows how to create and manipulate text frames
*/
protected void TextFrameExample ()
{
try
{
// Use the document's factory to create a new text frame and immediately access
// it's XTextFrame interface
XTextFrame xFrame = UnoRuntime.queryInterface (
XTextFrame.class, mxDocFactory.createInstance (
"com.sun.star.text.TextFrame" ) );
// Access the XShape interface of the TextFrame
XShape xShape = UnoRuntime.queryInterface(XShape.class, xFrame);
// Access the XPropertySet interface of the TextFrame
XPropertySet xFrameProps = UnoRuntime.queryInterface(
XPropertySet.class, xFrame );
// Set the size of the new Text Frame using the XShape's 'setSize' method
Size aSize = new Size();
aSize.Height = 400;
aSize.Width = 15000;
xShape.setSize(aSize);
// Set the AnchorType to com.sun.star.text.TextContentAnchorType.AS_CHARACTER
xFrameProps.setPropertyValue( "AnchorType", TextContentAnchorType.AS_CHARACTER );
// Go to the end of the text document
mxDocCursor.gotoEnd( false );
// Insert a new paragraph
mxDocText.insertControlCharacter (
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false );
// Then insert the new frame
mxDocText.insertTextContent(mxDocCursor, xFrame, false);
// Access the XText interface of the text contained within the frame
XText xFrameText = xFrame.getText();
// Create a TextCursor over the frame's contents
XTextCursor xFrameCursor = xFrameText.createTextCursor();
// Insert some text into the frame
xFrameText.insertString(
xFrameCursor, "The first line in the newly created text frame.", false );
xFrameText.insertString(
xFrameCursor, "\nThe second line in the new text frame.", false );
// Insert a paragraph break into the document (not the frame)
mxDocText.insertControlCharacter (
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false );
}
catch (Exception e)
{
e.printStackTrace ( System.out );
}
}
Embedded Objects
A TextEmbeddedObject
is a com.sun.star.text.BaseFrame providing the interface com.sun.star.document.XEmbeddedObjectSupplier. The only method of this interface,
com::sun::star::lang::XComponent getEmbeddedObject ()
provides access to the model of the embedded document. That way, an embedded LibreOffice spreadsheet, drawing, chart or a formula document can be used in a text over its document model.
An embedded object is inserted by using the document's factory to create an instance of the the service com.sun.star.text.TextEmbeddedObject. The type of object is determined by setting the string property CLSID to an appropriate value before inserting the object as text content in the document.
///***************************************************************************
// comment: Step 1: get the Desktop object from the office
// Step 2: open an empty text document
// Step 3: insert a sample text table
// Step 4: insert a Chart object
// Step 5: insert data from text table into Chart object
//***************************************************************************
import com.sun.star.uno.UnoRuntime;
public class OleObject {
public static void main(String args[]) {
// You need the desktop to create a document
// The getDesktop method does the UNO bootstrapping, gets the
// remote servie manager and the desktop object.
com.sun.star.frame.XDesktop xDesktop = null;
xDesktop = getDesktop();
com.sun.star.text.XTextDocument xTextDocument =
createTextdocument( xDesktop );
com.sun.star.text.XTextTable xTextTable =
createExampleTable( xTextDocument );
try {
// create TextEmbeddedObject
com.sun.star.lang.XMultiServiceFactory xDocMSF = (com.sun.star.lang.XMultiServiceFactory)
UnoRuntime.queryInterface(com.sun.star.lang.XMultiServiceFactory.class, xTextDocument);
com.sun.star.text.XTextContent xObj = (com.sun.star.text.XTextContent)
UnoRuntime.queryInterface(com.sun.star.text.XTextContent.class,
xDocMSF.createInstance( "com.sun.star.text.TextEmbeddedObject" ));
// set class id for chart object to determine the type
// of object to be inserted
com.sun.star.beans.XPropertySet xPS = (com.sun.star.beans.XPropertySet)
UnoRuntime.queryInterface(com.sun.star.beans.XPropertySet.class, xObj);
xPS.setPropertyValue( "CLSID", "12dcae26-281f-416f-a234-c3086127382e" );
// insert object in document
com.sun.star.text.XTextCursor xCursor = xTextDocument.getText().createTextCursor();
com.sun.star.text.XTextRange xRange = (com.sun.star.text.XTextRange)
UnoRuntime.queryInterface(com.sun.star.text.XTextRange.class, xCursor);
xTextDocument.getText().insertTextContent( xRange, xObj, false );
// access objects model
com.sun.star.document.XEmbeddedObjectSupplier xEOS = (com.sun.star.document.XEmbeddedObjectSupplier)
UnoRuntime.queryInterface(com.sun.star.document.XEmbeddedObjectSupplier.class, xObj);
com.sun.star.lang.XComponent xModel = xEOS.getEmbeddedObject();
// get table data
com.sun.star.chart.XChartDataArray xDocCDA = (com.sun.star.chart.XChartDataArray)
UnoRuntime.queryInterface(com.sun.star.chart.XChartDataArray.class, xTextTable);
double[][] aData = xDocCDA.getData();
// insert table data in Chart object
com.sun.star.chart.XChartDocument xChartDoc = (com.sun.star.chart.XChartDocument)
UnoRuntime.queryInterface(com.sun.star.chart.XChartDocument.class, xModel);
com.sun.star.chart.XChartDataArray xChartDataArray = (com.sun.star.chart.XChartDataArray)
UnoRuntime.queryInterface(com.sun.star.chart.XChartDataArray.class, xChartDoc.getData());
xChartDataArray.setData( aData );
// to remove the embedded object just uncomment the next line
//xTextDocument.getText().removeTextContent( xObj );
}
catch( Exception e) {
e.printStackTrace(System.err);
}
System.out.println("Done");
System.exit(0);
}
protected static com.sun.star.text.XTextTable createExampleTable(
com.sun.star.text.XTextDocument xTextDocument )
{
com.sun.star.lang.XMultiServiceFactory xDocMSF =
UnoRuntime.queryInterface(
com.sun.star.lang.XMultiServiceFactory.class, xTextDocument);
com.sun.star.text.XTextTable xTT = null;
try {
Object oInt = xDocMSF.createInstance("com.sun.star.text.TextTable");
xTT = (com.sun.star.text.XTextTable)
UnoRuntime.queryInterface(com.sun.star.text.XTextTable.class,oInt);
//initialize the text table with 4 columns an 5 rows
xTT.initialize(4,5);
} catch (Exception e) {
System.err.println("Couldn't create instance "+ e);
e.printStackTrace(System.err);
}
com.sun.star.text.XText xText = xTextDocument.getText();
//create a cursor object
com.sun.star.text.XTextCursor xTCursor = xText.createTextCursor();
//insert the table
try {
xText.insertTextContent(xTCursor, xTT, false);
} catch (Exception e) {
System.err.println("Couldn't insert the table " + e);
e.printStackTrace(System.err);
}
// inserting sample data
(xTT.getCellByName("A2")).setValue(5.0);
(xTT.getCellByName("A3")).setValue(5.5);
(xTT.getCellByName("A4")).setValue(5.7);
(xTT.getCellByName("B2")).setValue(2.3);
(xTT.getCellByName("B3")).setValue(2.2);
(xTT.getCellByName("B4")).setValue(2.4);
(xTT.getCellByName("C2")).setValue(6);
(xTT.getCellByName("C3")).setValue(6);
(xTT.getCellByName("C4")).setValue(6);
(xTT.getCellByName("D2")).setValue(3);
(xTT.getCellByName("D3")).setValue(3.5);
(xTT.getCellByName("D4")).setValue(4);
(xTT.getCellByName("E2")).setValue(8);
(xTT.getCellByName("E3")).setValue(5);
(xTT.getCellByName("E4")).setValue(3);
return xTT;
}
public static com.sun.star.frame.XDesktop getDesktop() {
com.sun.star.frame.XDesktop xDesktop = null;
com.sun.star.lang.XMultiComponentFactory xMCF = null;
try {
com.sun.star.uno.XComponentContext xContext = null;
// get the remote office component context
xContext = com.sun.star.comp.helper.Bootstrap.bootstrap();
// get the remote office service manager
xMCF = xContext.getServiceManager();
if( xMCF != null ) {
System.out.println("Connected to a running office ...");
Object oDesktop = xMCF.createInstanceWithContext(
"com.sun.star.frame.Desktop", xContext);
xDesktop = UnoRuntime.queryInterface(
com.sun.star.frame.XDesktop.class, oDesktop);
}
else
System.out.println( "Can't create a desktop. No connection, no remote office servicemanager available!" );
}
catch( Exception e) {
e.printStackTrace(System.err);
System.exit(1);
}
return xDesktop;
}
public static com.sun.star.text.XTextDocument createTextdocument(
com.sun.star.frame.XDesktop xDesktop )
{
com.sun.star.text.XTextDocument aTextDocument = null;
try {
com.sun.star.lang.XComponent xComponent = CreateNewDocument(xDesktop, "swriter");
aTextDocument = (com.sun.star.text.XTextDocument)
UnoRuntime.queryInterface(
com.sun.star.text.XTextDocument.class, xComponent);
}
catch( Exception e) {
e.printStackTrace(System.err);
}
return aTextDocument;
}
protected static com.sun.star.lang.XComponent CreateNewDocument(
com.sun.star.frame.XDesktop xDesktop,
String sDocumentType )
{
String sURL = "private:factory/" + sDocumentType;
com.sun.star.lang.XComponent xComponent = null;
com.sun.star.frame.XComponentLoader xComponentLoader = null;
com.sun.star.beans.PropertyValue xValues[] =
new com.sun.star.beans.PropertyValue[1];
com.sun.star.beans.PropertyValue xEmptyArgs[] =
new com.sun.star.beans.PropertyValue[0];
try {
xComponentLoader = (com.sun.star.frame.XComponentLoader)
UnoRuntime.queryInterface(
com.sun.star.frame.XComponentLoader.class, xDesktop);
xComponent = xComponentLoader.loadComponentFromURL(
sURL, "_blank", 0, xEmptyArgs);
}
catch( Exception e) {
e.printStackTrace(System.err);
}
return xComponent ;
}
}
Graphic Objects
A TextGraphicObject
is a BaseFrame
and does not provide any additional interfaces, compared with com.sun.star.text.BaseFrame. However, it introduces a number of properties that allow manipulating of a graphic object. They are described in the service com.sun.star.text.TextGraphicObject:
Properties of com.sun.star.text.TextGraphicObject | |
---|---|
ImageMap | com.sun.star.container.XIndexContainer. Returns the client-side image map if one is assigned to the object. |
ContentProtected | boolean - Determines if the content is protected against changes from the user interface.
|
SurroundContour | boolean - Determines if the text wraps around the contour of the object.
|
ContourOutside | boolean - The text flows only around the contour of the object.
|
ContourPolyPolygon | [optional] struct com.sun.star.drawing.PointSequenceSequence. Contains the contour of the object as PolyPolygon.
|
GraphicCrop | struct com.sun.star.text.GraphicCrop. Contains the cropping of the object.
|
HoriMirroredOnEvenPages | boolean - Determines if the object is horizontally mirrored on even pages.
|
HoriMirroredOnOddPages | boolean - Determines if the object is horizontally mirrored on odd pages.
|
VertMirrored | boolean - Determines if the object is mirrored vertically.
|
GraphicURL | string - Contains the URL of the background graphic of the object.
|
GraphicFilter | string - Contains the name of the filter of the background graphic of the object.
|
ActualSize | com.sun.star.awt.Size. Contains the original size of the bitmap in the graphic object. |
AdjustLuminance | short - Changes the display of the luminance. It contains percentage values between -100 and +100.
|
AdjustContrast | short - Changes the display of contrast. It contains percentage values between -100 and +100.
|
AdjustRed | short - Changes the display of the red color channel. It contains percentage values between -100 and +100.
|
AdjustGreen | short - Changes the display of the green color channel. It contains percentage values between -100 and +100.
|
AdjustBlue | short - Changes the display of the blue color channel. It contains percentage values between -100 and +100.
|
Gamma | double - Determines the gamma value of the graphic.
|
GraphicIsInverted | boolean - Determines if the graphic is displayed in inverted colors. It contains percentage values between -100 and +100.
|
Transparency | short - Measure of transparency. It contains percentage values between -100 and +100.
|
GraphicColorMode | long - Contains the ColorMode according to com.sun.star.drawing.ColorMode.
|
Drawing Shapes
Writer uses the same drawing engine as LibreOffice Impress and LibreOffice Draw. The limitations are that in Writer only one drawpage can exist and 3D objects are not supported. All drawing shapes support these properties:
Properties of com.sun.star.drawing.Shape | |
---|---|
ZOrder | [optional] long - Is used to query or change the ZOrder of this Shape .
|
LayerID | [optional] short - This is the ID of the layer to which this shape is attached.
|
LayerName | [optional] string - This is the name of the layer to which this Shape is attached.
|
Printable | [optional] boolean - If this is false, the shape is not visible on printer outputs.
|
MoveProtect | [optional] boolean - When set to true, this shape cannot be moved interactively in the user interface.
|
Name | [optional] string - This is the name of this shape.
|
SizeProtect | [optional] boolean - When set to true, this shape may not be sized interactively in the user interface.
|
Style | [optional] com.sun.star.style.XStyle. Determines the style for this shape.
|
Transformation | [optional] com.sun.star.drawing.HomogenMatrix This property lets you get and set the transformation matrix for this shape. The transformation is a 3x3 blended matrix and can contain translation, rotation, shearing and scaling.
|
ShapeUserDefinedAttributes | [optional] com.sun.star.container.XNameContainer. This property stores xml attributes. They are saved to and restored from automatic styles inside xml files.
|
In addition to the properties of the shapes natively supported by the drawing engine, the writer shape adds some properties, so that they are usable for text documents. These are defined in the service com.sun.star.text.Shape:
Properties of com.sun.star.text.Shape | |
---|---|
AnchorPageNo | short - Contains the number of the page where the objects are anchored.
|
AnchorFrame | com.sun.star.text.XTextFrame. Contains the text frame the current frame is anchored to. |
SurroundAnchorOnly | boolean - Determines if the text of the paragraph in which the object is anchored, wraps around the object.
|
AnchorType | [optional] com.sun.star.text.TextContentAnchorType. Specifies how the text content is attached to its surrounding text.
|
HoriOrient | short - Determines the horizontal orientation of the object.
|
HoriOrientPosition | long - Contains the horizontal position of the object (1/100 mm).
|
HoriOrientRelation | short - Determines the environment of the object to which the orientation is related.
|
VertOrient | short - Determines the vertical orientation of the object.
|
VertOrientPosition | long - Contains the vertical position of the object (1/100 mm). Valid only if TextEmbeddedObject::VertOrient is VertOrientation::NONE .
|
VertOrientRelation | short - Determines the environment of the object to which the orientation is related.
|
LeftMargin | long - Contains the left margin of the object.
|
RightMargin | long - Contains the right margin of the object.
|
TopMargin | long - Contains the top margin of the object.
|
BottomMargin | long - Contains the bottom margin of the object.
|
Surround | [deprecated] . Determines the type of the surrounding text.
|
SurroundAnchorOnly | boolean - Determines if the text of the paragraph in which the object is anchored, wraps around the object.
|
SurroundContour | boolean - Determines if the text wraps around the contour of the object.
|
ContourOutside | boolean - The text flows only around the contour of the object.
|
Opaque | boolean - Determines if the object is opaque or transparent for text.
|
TextRange | com.sun.star.text.XTextRange. Contains a text range where the shape should be anchored to. |
The chapter Drawing Documents and Presentation Documents describes how to use shapes and the interface of the draw page.
A sample that creates and inserts drawing shapes:
/** This method demonstrates how to create and manipulate shapes, and how to access the draw page
of the document to insert shapes
*/
protected void DrawPageExample () {
try {
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Insert two new paragraphs
mxDocText.insertControlCharacter(mxDocCursor,
ControlCharacter.PARAGRAPH_BREAK, false);
mxDocText.insertControlCharacter(mxDocCursor,
ControlCharacter.PARAGRAPH_BREAK, false);
// Get the XParagraphCursor interface of our document cursor
XParagraphCursor xParaCursor = (XParagraphCursor)
UnoRuntime.queryInterface(XParagraphCursor.class, mxDocCursor);
// Position the cursor in the 2nd paragraph
xParaCursor.gotoPreviousParagraph(false);
// Create a RectangleShape using the document factory
XShape xRect = UnoRuntime.queryInterface(
XShape.class, mxDocFactory.createInstance(
"com.sun.star.drawing.RectangleShape"));
// Create an EllipseShape using the document factory
XShape xEllipse = UnoRuntime.queryInterface(
XShape.class, mxDocFactory.createInstance(
"com.sun.star.drawing.EllipseShape"));
// Set the size of both the ellipse and the rectangle
Size aSize = new Size();
aSize.Height = 4000;
aSize.Width = 10000;
xRect.setSize(aSize);
aSize.Height = 3000;
aSize.Width = 6000;
xEllipse.setSize(aSize);
// Set the position of the Rectangle to the right of the ellipse
Point aPoint = new Point();
aPoint.X = 6100;
aPoint.Y = 0;
xRect.setPosition (aPoint);
// Get the XPropertySet interfaces of both shapes
XPropertySet xRectProps = UnoRuntime.queryInterface(
XPropertySet.class, xRect);
XPropertySet xEllipseProps = UnoRuntime.queryInterface(
XPropertySet.class, xEllipse);
// And set the AnchorTypes of both shapes to 'AT_PARAGRAPH'
xRectProps.setPropertyValue("AnchorType", TextContentAnchorType.AT_PARAGRAPH);
xEllipseProps.setPropertyValue("AnchorType", TextContentAnchorType.AT_PARAGRAPH);
// Access the XDrawPageSupplier interface of the document
XDrawPageSupplier xDrawPageSupplier = UnoRuntime.queryInterface(
XDrawPageSupplier.class, mxDoc);
// Get the XShapes interface of the draw page
XShapes xShapes = UnoRuntime.queryInterface(
XShapes.class, xDrawPageSupplier.getDrawPage());
// Add both shapes
xShapes.add (xEllipse);
xShapes.add (xRect);
/*
This doesn't work, I am assured that FME and AMA are fixing it.
XShapes xGrouper = (XShapes) UnoRuntime.queryInterface(
XShapes.class, mxDocFactory.createInstance(
"com.sun.star.drawing.GroupShape"));
XShape xGrouperShape = (XShape) UnoRuntime.queryInterface(XShape.class, xGrouper);
xShapes.add (xGrouperShape);
xGrouper.add (xRect);
xGrouper.add (xEllipse);
XShapeGrouper xShapeGrouper = UnoRuntime.queryInterface(
XShapeGrouper.class, xShapes);
xShapeGrouper.group (xGrouper);
*/
} catch (Exception e) {
e.printStackTrace(System.err);
}
}
Redline
Redlines are text portions created in the user interface by switching on com.sun.star.document.XRedlinesSupplier interface at the document model. A collection of redlines as com.sun.star.beans.XPropertySet objects are received that can be accessed as com.sun.star.container.XIndexAccess or as com.sun.star.container.XEnumerationAccess. Their properties are described in com.sun.star.text.RedlinePortion.
▸ ▸ . Redlines in a document are accessed through theIf a change is recorded, but not visible because the option RedlineText
, which is a com.sun.star.text.XText.
Calling XPropertySet.getPropertySetInfo()
on a redline property set crashes the office.
Ruby
Ruby text is a character layout attribute used in Asian languages. Ruby text appears above or below text in left to right writing, and left to right of text in top to bottom writing. For examples, cf. https://www.w3.org/TR/1999/WD-ruby-19990322/.
Ruby text is created using the appropriate character properties from the service com.sun.star.style.CharacterProperties wherever this service is supported. However, the Asian languages support must be switched on in Tools - Options - LanguageSettings - Languages.
There is no convenient supplier interface for ruby text at the model at this time. However, the controller has an interface com.sun.star.text.XRubySelection that provides access to rubies contained in the current selection.
To find ruby text in the model, enumerate all text portions in all paragraphs and check if the property TextPortionType
contains the string "Ruby
" to find ruby text. When there is ruby text, access the RubyText
property of the text portion that contains ruby text as a string.
CharacterProperties for Ruby Text | Description |
---|---|
RubyText | Contains the text that is set as ruby. |
RubyAdjust | Determines the adjustment of the ruby text as RubyAdjust .
|
RubyCharStyleName | Contains the name of the character style that is applied to RubyText .
|
RubyIsAbove | Determines if the ruby text is printed above/left or below/right of the text |
Overall Document Features
Styles
Styles distinguish sections in a document that are commonly formatted and separates this information from the actual formatting. This way it is possible to unify the appearance of a document, and adjust the formatting of a document by altering a style, instead of local format settings after the document has been completed. Styles are packages of attributes that can be applied to text or text contents in a single step.
The following style families are available in LibreOffice.
Style Families | Description |
---|---|
CharacterStyles | Character styles are used to format single characters or entire words and phrases. Character styles can be nested. |
ParagraphStyles | Paragraph styles are used to format entire paragraphs. Apart from the normal format settings for paragraphs, the paragraph style also defines the font to be used, and the paragraph style for the following paragraph. |
FrameStyles | Frame styles are used to format graphic and text frames. These Styles are used to quickly format graphics and frames automatically. |
PageStyles | Page styles are used to structure the page. If a "Next Style" is specified, the LibreOffice automatically applies the specified page style when an automatic page break occurs. |
NumberingStyles | Numbering styles are used to format paragraphs in numbered or bulleted text. |
The text document model implements the interface com.sun.star.style.XStyleFamiliesSupplier to access these styles. Its method getStyleFamilies()
returns a collection of com.sun.star.style.StyleFamilies with a com.sun.star.container.XNameAccess interface. The com.sun.star.container.XNameAccess interface retrieves the style families by the names listed above. The StyleFamilies
service supports a com.sun.star.container.XIndexAccess.
From the StyleFamilies
, retrieve one of the families listed above by name or index. A collection of styles are received which is a com.sun.star.style.StyleFamily service, providing access to the single styles through an com.sun.star.container.XNameContainer or an com.sun.star.container.XIndexAccess.
Each style is a com.sun.star.style.Style and supports the interface com.sun.star.style.XStyle that inherits from com.sun.star.container.XNamed. The XStyle
contains:
string getName() void setName( [in] string aName) boolean isUserDefined() boolean isInUse() string getParentStyle() void setParentStyle( [in] string aParentStyle)
The office comes with a set of default styles. These styles use programmatic names on the API level. The method setName()
in XStyle
always throws an exception if called at such styles. The same applies to changing the property Category. At the user interface localized names are used. The user interface names are provided through the property UserInterfaceName
.
Note that page and numbering styles are not hierarchical and cannot have parent styles. The method getParentStyle()
always returns an empty string, and the method setParentStyle()
throws a com.sun.star.uno.RuntimeException when called at a default style.
The method isUserDefined()
determines whether a style is defined by a user or is a built-in style. A built-in style cannot be deleted. Additionally the built-in styles have two different names: a true object name and an alias that is displayed at the user interface. This is not usually visible in an English LibreOffice version, except for the default styles that are named "Standard" as programmatic name and "Default" in the user interface.
The Style service defines the following properties which are shared by all styles:
Properties of com.sun.star.style.Style | |
---|---|
IsPhysical | [optional, readonly] boolean - Determines if a style is physically created.
|
FollowStyle | [optional] boolean - Contains the name of the style that is applied to the next paragraph.
|
DisplayName | [optional, readonly] string - Contains the name of the style as is displayed in the user interface.
|
IsAutoUpdate | [optional] string - Determines if a style is automatically updated when the properties of an object that the style is applied to are changed.
|
ParaStyleConditions | [optional, property] sequence< com.sun.star.beans.NamedValue > - Defines the context and styles for conditional paragraphs. This property is only available if the style is a conditional paragraph style.
|
To determine the user interface name, each style has a string property DisplayName
that contains the name that is used at the user interface. It is not allowed to use a DisplayName
of a style as a name of a user-defined style of the same style family.
The built-in styles are not created actually as long as they are not used in the document. The property IsPhysical
checks for this. It is necessary, for file export purposes, to detect styles which do not need to be exported.
Conditional paragraph styles are handled by the property ParaStyleConditions. The sequence consists of pairs where the name part (the first part) of the pair defines the context where the style (the second part, a string that denotes a style name or an empty string) should be applied to. Assigning an empty string to the style name will disable the conditional style for that context.
The StyleFamilies
collection can load styles. For this purpose, the interface com.sun.star.style.XStyleLoader is available at the StyleFamilies
collection. It consists of two methods:
void loadStylesFromURL( [in] string URL, [in] sequence< com::sun::star::beans::PropertyValue > aOptions) sequence< com::sun::star::beans::PropertyValue > getStyleLoaderOptions()
The method loadStylesFromURL()
enables the document to import styles from other documents. The expected sequence of PropertyValue
structs can contain the following properties:
Properties for loadStylesFromURL() | Description |
---|---|
LoadTextStyles
|
Determines if character and paragraph styles are to be imported. It is not possible to select character styles and paragraph styles separately. |
LoadFrameStyles
|
boolean - Import frame styles only.
|
LoadPageStyles
|
boolean - Import page styles only.
|
LoadNumberingStyles
|
boolean - Import numbering styles only.
|
OverwriteStyles
|
boolean - Determines if internal styles are overwritten if the source document contains styles having the same name.
|
The method getStyleLoaderOptions()
returns a sequence of these PropertyValue
structs, set to their default values.
Character Styles
Character styles support all properties defined in the services com.sun.star.style.CharacterProperties and com.sun.star.style.CharacterPropertiesAsian.
They are created using the com.sun.star.lang.XMultiServiceFactory interface of the text document model using the service name "com.sun.star.style.CharacterStyle
".
The default style that is shown in the user interface and accessible through the API is not a style, but a tool to remove applied character styles. Therefore, its properties cannot be changed.
Set the property CharStyleName
at an object including the service com.sun.star.style.CharacterProperties to set its character style.
Paragraph Styles
Paragraph styles support all properties defined in the services com.sun.star.style.ParagraphProperties and com.sun.star.style.ParagraphPropertiesAsian.
They are created using the com.sun.star.lang.XMultiServiceFactory interface of the text document model using the service name "com.sun.star.style.ParagraphStyle
".
Additionally, there is a service com.sun.star.style.ConditionalParagraphStyle which creates conditional paragraph styles. Conditional styles are paragraph styles that have different effects, depending on the context. There is currently no support of the condition properties at the API.
Set the property ParaStyleName
at an object, including the service com.sun.star.style.ParagraphProperties to set its paragraph style.
Frame Styles
Frame styles support all properties defined in the services com.sun.star.text.BaseFrameProperties.
The frame styles are applied to text frames, graphic objects and embedded objects.
They are created using the com.sun.star.lang.XMultiServiceFactory interface of the text document model using the service name "com.sun.star.style.FrameStyle
".
Set the property FrameStyleName
at com.sun.star.text.BaseFrame objects to set their frame style.
Page Styles
Page styles are controlled via properties. The page related properties are defined in the services com.sun.star.style.PageStyle
They are created using the com.sun.star.lang.XMultiServiceFactory interface of the text document model using the service name "com.sun.star.style.PageStyle
".
As mentioned above, page styles are not hierarchical. The section Page Layout discusses page styles.
The PageStyle
is set at the current text cursor position by setting the property PageDescName
to an existing page style name.This will insert a new page that uses the new page style. If no new page should be inserted, the cursor has to be at the beginning of the first paragraph.
Numbering Styles
Numbering styles support all properties defined in the services com.sun.star.text.NumberingStyle.
They are created using the com.sun.star.lang.XMultiServiceFactory interface of the text document model using the service name "com.sun.star.style.NumberingStyle
".
The structure of the numbering rules is described in section Line Numbering and Outline Numbering.
The name of the numbering style is set in the property NumberingStyleName
of paragraphs (set through the PropertySet
of a TextCursor
) or a paragraph style to apply the numbering to the paragraphs.
The following example demonstrates the use of paragraph styles:
/** This method demonstrates how to create, insert and apply styles
*/
protected void StylesExample() {
try {
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Insert two paragraph breaks
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
// Create a new style from the document's factory
XStyle xStyle = UnoRuntime.queryInterface(
XStyle.class, mxDocFactory.createInstance("com.sun.star.style.ParagraphStyle"));
// Access the XPropertySet interface of the new style
XPropertySet xStyleProps = UnoRuntime.queryInterface(
XPropertySet.class, xStyle);
// Give the new style a light blue background
xStyleProps.setPropertyValue ("ParaBackColor", Integer.valueOf(13421823));
// Get the StyleFamiliesSupplier interface of the document
XStyleFamiliesSupplier xSupplier = UnoRuntime.queryInterface(
XStyleFamiliesSupplier.class, mxDoc);
// Use the StyleFamiliesSupplier interface to get the XNameAccess interface of the
// actual style families
XNameAccess xFamilies = UnoRuntime.queryInterface (
XNameAccess.class, xSupplier.getStyleFamilies());
// Access the 'ParagraphStyles' Family
XNameContainer xFamily = UnoRuntime.queryInterface(
XNameContainer.class, xFamilies.getByName("ParagraphStyles"));
// Insert the newly created style into the ParagraphStyles family
xFamily.insertByName ("All-Singing All-Dancing Style", xStyle);
// Get the XParagraphCursor interface of the document cursor
XParagraphCursor xParaCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, mxDocCursor);
// Select the first paragraph inserted
xParaCursor.gotoPreviousParagraph(false);
xParaCursor.gotoPreviousParagraph(true);
// Access the property set of the cursor selection
XPropertySet xCursorProps = UnoRuntime.queryInterface(
XPropertySet.class, mxDocCursor);
// Set the style of the cursor selection to our newly created style
xCursorProps.setPropertyValue("ParaStyleName", "All-Singing All-Dancing Style");
// Go back to the end
mxDocCursor.gotoEnd(false);
// Select the last paragraph in the document
xParaCursor.gotoNextParagraph(true);
// And reset it's style to 'Standard' (the programmatic name for the default style)
xCursorProps.setPropertyValue("ParaStyleName", "Standard");
} catch (Exception e) {
e.printStackTrace (System.out);
}
}
Settings
General Document Information
Text documents offer general information about the document through their com.sun.star.document.XDocumentPropertiesSupplier interface. The DocumentProperties is a common LibreOffice feature and is discussed in Office Development.
The XDocumentPropertiesSupplier has one single method:
com::sun::star::document::XDocumentProperties getDocumentProperties()
which returns a com.sun.star.document.DocumentProperties service, offering metadata and statistical information about the document that is available through File - Properties in the GUI.
Document Properties
The model implements a com.sun.star.beans.XPropertySet that provides properties concerning character formatting and general settings.
The properties for character attributes are CharFontName
, CharFontStyleName
, CharFontFamily
, CharFontCharSet
, CharFontPitch
and their Asian counterparts CharFontStyleNameAsian
, CharFontFamilyAsian
, CharFontCharSetAsian
, CharFontPitchAsian
.
The following properties handle general settings:
Properties of com.sun.star.text.TextDocument | |
---|---|
CharLocale | com.sun.star.lang.Locale. Default locale of the document. |
CharacterCount | long - Number of characters.
|
ParagraphCount | long - Number of paragraphs.
|
WordCount | long - Number of words.
|
WordSeparator | string - Contains all that characters that are treated as separators between words to determine word count.
|
RedlineDisplayType | short - Displays redlines as defined in com.sun.star.document.RedlineDisplayType.
|
RecordChanges | boolean - Determines if redlining is switched on.
|
ShowChanges | boolean - Determines if redlines are displayed.
|
RedlineProtectionKey | sequence < byte > . Contains the password key.
|
ForbiddenCharacters | com.sun.star.i18n.ForbiddenCharacters. Contains characters that are not allowed to be at the first or last character of a text line. |
TwoDigitYear | short - Determines the start of the range, for example, when entering a two-digit year.
|
IndexAutoMarkFileURL | string - The URL to the file that contains the search words and settings for the automatic marking of index marks for alphabetical indexes.
|
AutomaticControlFocus | boolean - If true, the first form object is selected when the document is loaded.
|
ApplyFormDesignMode | boolean - Determines if form (database) controls are in the design mode.
|
HideFieldTips | boolean - If true, the automatic tips displayed for some types of text fields are suppressed.
|
Creating Default Settings
The com.sun.star.lang.XMultiServiceFactory implemented at the model provides the service com.sun.star.text.Defaults. Use this service to find default values to set paragraph and character properties of the document to default.
Creating Document Settings
Another set of properties can be created by the service name com.sun.star.document.Settings that contains a number of additional settings.
Line Numbering and Outline Numbering
LibreOffice provides automatic numbering for texts. For instance, paragraphs can be numbered or listed with bullets in a hierarchical manner, chapter headings can be numbered and lines can be counted and numbered.
Paragraph and Outline Numbering
com.sun.star.text.NumberingRulesThe key for paragraph numbering is the paragraph property NumberingRules
. This property is provided by paragraphs and numbering styles and is a member of com.sun.star.style.ParagraphProperties.
A similar object controls outline numbering and is returned from the method:
com::sun::star::container::XIndexReplace getChapterNumberingRules()
at the com.sun.star.text.XChapterNumberingSupplier interface that is implemented at the document model.
These objects provide an interface com.sun.star.container.XIndexReplace. Each element of the container represents a numbering level. The writer document provides ten numbering levels. The highest level is zero. Each level of the container consists of a sequence of com.sun.star.beans.PropertyValue.
The two related objects differ in some of properties they provide.
Both of them provide the following properties:
Common Properties for Paragraph and Outline Numbering in com.sun.star.text.NumberingLevel | |
---|---|
Adjust | short - Adjustment of the numbering symbol defined in com.sun.star.text.HoriOrientation.
|
ParentNumbering | short - Determines if higher numbering levels are included in the numbering, for example, 2.3.1.2.
|
Prefix |
string - Contains strings that surround the numbering symbol, for example, brackets.
|
CharStyleName | string - Name of the character style that is applied to the number symbol.
|
StartWith | short - Determines the value the numbering starts with. The default is one.
|
FirstLineOffset |
long - Influences the left indent and left margin of the numbering.
|
SymbolTextDistance | [optional] long - Distance between the numbering symbol and the text of the paragraph.
|
NumberingType | short - Determines the type of the numbering defined in com.sun.star.style.NumberingType.
|
Only paragraphs have the following properties in their NumberingRules
property:
Paragraph NumberingRules Properties in com.sun.star.text.NumberingLevel | Description |
---|---|
BulletChar | string - Determines the bullet character if the numbering type is set to NumberingType::CHAR_SPECIAL .
|
BulletFontName | string - Determines the bullet font if the numbering type is set to NumberingType::CHAR_SPECIAL .
|
GraphicURL | string - Determines the type, size and orientation of a graphic when the numbering type is set to NumberingType::BITMAP .
|
GraphicBitmap | com.sun.star.awt.XBitmap - the bitmap containing the bullet. |
GraphicSize | com.sun.star.awt.Size - size of the graphic that is used as bullet. |
VertOrient | short - Vertical orientation of a graphic according to com.sun.star.text.VertOrientation
|
Only the chapter numbering rules provide the following property:
Property of com.sun.star.text.ChapterNumberingRule | Description |
---|---|
HeadingStyleName | string - Contains the name of the paragraph style that marks a paragraph as a chapter heading.
|
The following is an example for the NumberingRules
service:
/** This method demonstrates how to set numbering types and numbering levels using the
com.sun.star.text.NumberingRules service
*/
protected void NumberingExample() {
try {
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Get the RelativeTextContentInsert interface of the document
XRelativeTextContentInsert xRelative = (XRelativeTextContentInsert)
UnoRuntime.queryInterface(XRelativeTextContentInsert.class, mxDocText);
// Use the document's factory to create the NumberingRules service, and get it's
// XIndexAccess interface
XIndexAccess xNum = UnoRuntime.queryInterface(XIndexAccess.class,
mxDocFactory.createInstance("com.sun.star.text.NumberingRules"));
// Also get the NumberingRule's XIndexReplace interface
XIndexReplace xReplace = UnoRuntime.queryInterface(
XIndexReplace.class, xNum);
// Create an array of XPropertySets, one for each of the three paragraphs we're about
// to create
XPropertySet xParas[] = new XPropertySet[3];
for (int i = 0 ; i < 3 ; ++ i) {
// Create a new paragraph
XTextContent xNewPara = UnoRuntime.queryInterface(
XTextContent.class, mxDocFactory.createInstance(
"com.sun.star.text.Paragraph"));
// Get the XPropertySet interface of the new paragraph and put it in our array
xParas[i] = UnoRuntime.queryInterface(
XPropertySet.class, xNewPara);
// Insert the new paragraph into the document after the fish section. As it is
// an insert
// relative to the fish section, the first paragraph inserted will be below
// the next two
xRelative.insertTextContentAfter (xNewPara, mxFishSection);
// Separate from the above, but also needs to be done three times
// Get the PropertyValue sequence for this numbering level
PropertyValue[] aProps = (PropertyValue []) xNum.getByIndex(i);
// Iterate over the PropertyValue's for this numbering level, looking for the
// 'NumberingType' property
for (int j = 0 ; j < aProps.length ; ++j) {
if (aProps[j].Name.equals ("NumberingType")) {
// Once we find it, set it's value to a new type,
// dependent on which
// numbering level we're currently on
switch ( i ) {
case 0 : aProps[j].Value = new Short(NumberingType.ROMAN_UPPER);
break;
case 1 : aProps[j].Value = new Short(NumberingType.CHARS_UPPER_LETTER);
break;
case 2 : aProps[j].Value = new Short(NumberingType.ARABIC);
break;
}
// Put the updated PropertyValue sequence back into the
// NumberingRules service
xReplace.replaceByIndex (i, aProps);
break;
}
}
}
// Get the XParagraphCursor interface of our text cursor
XParagraphCursor xParaCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, mxDocCursor);
// Go to the end of the document, then select the preceding paragraphs
mxDocCursor.gotoEnd(false);
xParaCursor.gotoPreviousParagraph false);
xParaCursor.gotoPreviousParagraph true);
xParaCursor.gotoPreviousParagraph true);
// Get the XPropertySet of the cursor's currently selected text
XPropertySet xCursorProps = UnoRuntime.queryInterface(
XPropertySet.class, mxDocCursor);
// Set the updated Numbering rules to the cursor's property set
xCursorProps.setPropertyValue ("NumberingRules", xNum);
mxDocCursor.gotoEnd(false);
// Set the first paragraph that was inserted to a numbering level of 2 (thus it will
// have Arabic style numbering)
xParas[0].setPropertyValue ("NumberingLevel", new Short ((short) 2));
// Set the second paragraph that was inserted to a numbering level of 1 (thus it will
// have 'Chars Upper Letter' style numbering)
xParas[1].setPropertyValue ("NumberingLevel", new Short((short) 1));
// Set the third paragraph that was inserted to a numbering level of 0 (thus it will
// have 'Roman Upper' style numbering)
xParas[2].setPropertyValue("NumberingLevel", new Short((short) 0));
} catch (Exception e) {
e.printStackTrace (System.out);
}
}
Line Numbering
The text document model supports the interface com.sun.star.text.XLineNumberingProperties. The provided object has the properties described in the service com.sun.star.text.LineNumberingProperties. It is used in conjunction with the paragraph properties ParaLineNumberCount
and ParaLineNumberStartValue
.
Number Formats
The text document model provides access to the number formatter through aggregation, that is, it provides the interface com.sun.star.util.XNumberFormatsSupplier seamlessly.
The number formatter is used to format numerical values. For details, refer to Number Formats.
In text, text fields with numeric content and table cells provide a property NumberFormat
that contains a long value that refers to a number format.
Text Sections
A text section is a range of complete paragraphs that can have its own format settings and source location, separate from the surrounding text. Text sections can be nested in a hierarchical structure.
For example, a section is formatted to have text columns that different column settings in a text on a paragraph by paragraph basis. The content of a section can be linked through file links or over a DDE connection.
The text sections support the service com.sun.star.text.TextSection. To access the sections, the text document model implements the interface com.sun.star.text.XTextSectionsSupplier that provides an interface com.sun.star.container.XNameAccess. The returned objects support the interface com.sun.star.container.XIndexAccess, as well.
Master documents implement the structure of sub documents using linked text sections.
An example demonstrating the creation, insertion and linking of text sections:
/** This method demonstrates how to create linked and unlinked sections
*/
protected void TextSectionExample() {
try {
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Insert two paragraph breaks
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, true);
// Create a new TextSection from the document factory and access it's XNamed interface
XNamed xChildNamed = UnoRuntime.queryInterface(
XNamed.class, mxDocFactory.createInstance("com.sun.star.text.TextSection"));
// Set the new sections name to 'Child_Section'
xChildNamed.setName("Child_Section");
// Access the Child_Section's XTextContent interface and insert it into the document
XTextContent xChildSection = UnoRuntime.queryInterface(
XTextContent.class, xChildNamed);
mxDocText.insertTextContent (mxDocCursor, xChildSection, false);
// Access the XParagraphCursor interface of our text cursor
XParagraphCursor xParaCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, mxDocCursor);
// Go back one paragraph (into Child_Section)
xParaCursor.gotoPreviousParagraph(false);
// Insert a string into the Child_Section
mxDocText.insertString(mxDocCursor, "This is a test", false);
// Go to the end of the document
mxDocCursor.gotoEnd(false);
// Go back two paragraphs
xParaCursor.gotoPreviousParagraph (false);
xParaCursor.gotoPreviousParagraph (false);
// Go to the end of the document, selecting the two paragraphs
mxDocCursor.gotoEnd(true);
// Create another text section and access it's XNamed interface
XNamed xParentNamed = UnoRuntime.queryInterface(XNamed.class,
mxDocFactory.createInstance("com.sun.star.text.TextSection"));
// Set this text section's name to Parent_Section
xParentNamed.setName ("Parent_Section");
// Access the Parent_Section's XTextContent interface ...
XTextContent xParentSection = UnoRuntime.queryInterface(
XTextContent.class, xParentNamed);
// ...and insert it into the document
mxDocText.insertTextContent(mxDocCursor, xParentSection, false);
// Go to the end of the document
mxDocCursor.gotoEnd (false);
// Insert a new paragraph
mxDocText.insertControlCharacter(
mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
// And select the new paragraph
xParaCursor.gotoPreviousParagraph(true);
// Create a new Text Section and access it's XNamed interface
XNamed xLinkNamed = UnoRuntime.queryInterface(
XNamed.class, mxDocFactory.createInstance("com.sun.star.text.TextSection"));
// Set the new text section's name to Linked_Section
xLinkNamed.setName("Linked_Section");
// Access the Linked_Section's XTextContent interface
XTextContent xLinkedSection = UnoRuntime.queryInterface(
XTextContent.class, xLinkNamed);
// And insert the Linked_Section into the document
mxDocText.insertTextContent(mxDocCursor, xLinkedSection, false);
// Access the Linked_Section's XPropertySet interface
XPropertySet xLinkProps = UnoRuntime.queryInterface(
XPropertySet.class, xLinkNamed);
// Set the linked section to be linked to the Child_Section
xLinkProps.setPropertyValue("LinkRegion", "Child_Section");
// Access the XPropertySet interface of the Child_Section
XPropertySet xChildProps = UnoRuntime.queryInterface(
XPropertySet.class, xChildNamed);
// Set the Child_Section's background colour to blue
xChildProps.setPropertyValue("BackColor", Integer.valueOf(13421823));
// Refresh the document, so the linked section matches the Child_Section
XRefreshable xRefresh = UnoRuntime.queryInterface(
XRefreshable.class, mxDoc);
xRefresh.refresh();
} catch (Exception e) {
e.printStackTrace (System.out);
}
}
Page Layout
A page layout in LibreOffice is always a page style. A page can not be hard formatted. To change the current page layout, retrieve the current page style from the text cursor property PageStyleName
and get this page style from the StyleFamily PageStyles
.
Changes of the page layout happen through the properties described in com.sun.star.style.PageProperties. Refer to the API reference for details on all the possible properties, including the header and footer texts which are part of these properties.
As headers or footers are connected to a page style, the text objects are provided as properties of the style. Depending on the setting of the page layout, there is one header and footer text object per style available or there are two, a left and right header, and footer text:
The page layout of a page style can be equal on left and right pages, mirrored, or separate for right and left pages. This is controlled by the property PageStyleLayout
that expects values from the enum com.sun.star.style.PageStyleLayout. As long as left and right pages are equal, HeaderText
and HeaderRightText
are identical. The same applies to the footers.
The text objects in headers and footers are only available if headers or footers are switched on, using the properties HeaderIsOn
and FooterIsOn
.
Drawing objects cannot be inserted into headers or footers.
Columns
Text frames, text sections and page styles can be formatted to have columns. The width of columns is relative since the absolute width of the object is unknown in the model. The layout formatting is responsible for calculating the actual widths of the columns.
Columns are applied using the property TextColumns
. It expects a com.sun.star.text.TextColumns service that has to be created by the document factory. The interface com.sun.star.text.XTextColumns refines the characteristics of the text columns before applying the created TextColumns
service to the property TextColumns
.
Consider the following example to see how to work with text columns:
/** This method demonstrates the XTextColumns interface and how to insert a blank paragraph
using the XRelativeTextContentInsert interface
*/
protected void TextColumnsExample() {
try {
// Go to the end of the doucment
mxDocCursor.gotoEnd(false);
// insert a new paragraph
mxDocText.insertControlCharacter(mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
// insert the string 'I am a fish.' 100 times
for (int i = 0 ; i < 100 ; ++i) {
mxDocText.insertString(mxDocCursor, "I am a fish.", false);
}
// insert a paragraph break after the text
mxDocText.insertControlCharacter(mxDocCursor, ControlCharacter.PARAGRAPH_BREAK, false);
// Get the XParagraphCursor interface of our text cursor
XParagraphCursor xParaCursor = UnoRuntime.queryInterface(
XParagraphCursor.class, mxDocCursor);
// Jump back before all the text we just inserted
xParaCursor.gotoPreviousParagraph(false);
xParaCursor.gotoPreviousParagraph(false);
// Insert a string at the beginning of the block of text
mxDocText.insertString(mxDocCursor, "Fish section begins:", false);
// Then select all of the text
xParaCursor.gotoNextParagraph(true);
xParaCursor.gotoNextParagraph(true);
// Create a new text section and get it's XNamed interface
XNamed xSectionNamed = UnoRuntime.queryInterface(
XNamed.class, mxDocFactory.createInstance("com.sun.star.text.TextSection"));
// Set the name of our new section (appropiately) to 'Fish'
xSectionNamed.setName("Fish");
// Create the TextColumns service and get it's XTextColumns interface
XTextColumns xColumns = UnoRuntime.queryInterface(
XTextColumns.class, mxDocFactory.createInstance("com.sun.star.text.TextColumns"));
// We want three columns
xColumns.setColumnCount((short) 3);
// Get the TextColumns, and make the middle one narrow with a larger margin
// on the left than the right
TextColumn[] aSequence = xColumns.getColumns ();
aSequence[1].Width /= 2;
aSequence[1].LeftMargin = 350;
aSequence[1].RightMargin = 200;
// Set the updated TextColumns back to the XTextColumns
xColumns.setColumns(aSequence);
// Get the property set interface of our 'Fish' section
XPropertySet xSectionProps = UnoRuntime.queryInterface(
XPropertySet.class, xSectionNamed);
// Set the columns to the Text Section
xSectionProps.setPropertyValue("TextColumns", xColumns);
// Get the XTextContent interface of our 'Fish' section
mxFishSection = UnoRuntime.queryInterface(
XTextContent.class, xSectionNamed);
// Insert the 'Fish' section over the currently selected text
mxDocText.insertTextContent(mxDocCursor, mxFishSection, true);
// Get the wonderful XRelativeTextContentInsert interface
XRelativeTextContentInsert xRelative = (XRelativeTextContentInsert)
UnoRuntime.queryInterface(XRelativeTextContentInsert.class, mxDocText);
// Create a new empty paragraph and get it's XTextContent interface
XTextContent xNewPara = UnoRuntime.queryInterface(XTextContent.class,
mxDocFactory.createInstance("com.sun.star.text.Paragraph"));
// Insert the empty paragraph after the fish Text Section
xRelative.insertTextContentAfter(xNewPara, mxFishSection);
} catch (Exception e) {
e.printStackTrace(System.err);
}
}
The text columns property consists of com.sun.star.text.TextColumn structs. The Width elements of all structs in the TextColumns
sequence make up a sum, that is provided by the method getReferenceValue()
of the XTextColumns
interface. To determine the metric width of an actual column, the reference value and the columns width element have to be calculated using the metric width of the object (page, text frame, text section) and a rule of three, for example:
nColumn3Width = aColumns[3].Width / xTextColumns.getReferenceValue() * RealObjectWidth
The column margins (LeftMargin
, and RightMargin
elements of the struct) are inside the column. Their values do not influence the column width. They just limit the space available for the column content.
The default column setting in LibreOffice creates columns with equal margins at inner columns, and no left margin at the leftmost column and no right margin at the rightmost column. Therefore, the relative width of the first and last column is smaller than those of the inner columns. This causes a limitation of this property: Setting the text columns with equal column content widths and equal margins is only possible when the width of the object (text frame, text section) can be determined. Unfortunately this is impossible when the width of the object depends on its environment itself.
Link targets
The interface com.sun.star.document.XLinkTargetSupplier of the document model provides all elements of the document that can be used as link targets. These targets can be used for load URLs and sets the selection to a certain position object inside of a document. An example of a URL containing a link target is "file:///c:/documents/document1|bookmarkname".
This interface is used from the hyperlink dialog to detect the links available inside of a document.
The interface com.sun.star.container.XNameAccess returned by the method getLinks()
provides access to an array of target types. These types are:
- Tables
- Text frame
- Graphics
- OLEObjects
- Sections
- Headings
- Bookmarks.
The names of the elements depend on the installed language.
Each returned object supports the interfaces com.sun.star.beans.XPropertySet and interface com.sun.star.container.XNameAccess. The property set provides the properties LinkDisplayName
(string) and LinkDisplayBitmap
(com.sun.star.awt.XBitmap). Each of these objects provides an array of targets of the relating type. Each target returned supports the interface com.sun.star.beans.XPropertySet and the property LinkDisplayName
(string).
The name of the objects is the bookmark to be added to the document URL, for example, "Table1|table". The LinkDisplayName
contains the name of the object, e.g. "Table1
".
Text Document Controller
The text document model knows its controller and it can lock the controller to block user interaction. The appropriate methods in the model's com.sun.star.frame.XModel interface are:
void lockControllers()
void unlockControllers()
boolean hasControllersLocked()
com::sun::star::frame::XController getCurrentController()
void setCurrentController( [in] com::sun::star::frame::XController xController)
The controller returned by getCurrentController()
shares the following interfaces with all other document controllers in LibreOffice:
- com.sun.star.frame.XController
- com.sun.star.frame.XDispatchProvider
- com.sun.star.ui.XContextMenuInterceptor
Document controllers are explained in the Office Development.
TextView
The writer controller implementation supports the interface com.sun.star.view.XSelectionSupplier that returns the object that is currently selected in the user interface.
Its method getSelection()
returns an any that may contain the following object depending on the selection:
Selection | Returned Object |
---|---|
Text | com.sun.star.container.XIndexAccess containing one or more com.sun.star.uno.XInterface pointing to a text range. |
Selection of table cells | com.sun.star.uno.XInterface pointing to a table cursor. |
Text frame | com.sun.star.uno.XInterface pointing to a text frame. |
Graphic object | com.sun.star.uno.XInterface pointing to a graphic object. |
OLE object | com.sun.star.uno.XInterface pointing to an OLE object. |
Shape, Form control | com.sun.star.uno.XInterface pointing to a com.sun.star.drawing.ShapeCollection containing one or more shapes. |
- provides access to the controller of form controls.
- provides access to the cursor of the view.
- provides access to rubies contained in the selection. This interface is necessary for Asian language support.
- provides access to the settings of the view as described in the service com.sun.star.text.ViewSettings.
Properties of com.sun.star.text.ViewSettings | |
---|---|
ShowAnnotations | boolean - If true, annotations (notes) are visible.
|
ShowBreaks | boolean - If true, paragraph line breaks are visible.
|
ShowDrawings | boolean - If true, shapes are visible.
|
ShowFieldCommands | boolean - If true, text fields are shown with their commands, otherwise the content is visible.
|
ShowFootnoteBackground | boolean - If true, footnotes symbols are displayed with gray background.
|
ShowGraphics | boolean - If true, graphic objects are visible.
|
ShowHiddenParagraphs | boolean - If true, hidden paragraphs are displayed.
|
ShowHiddenText | boolean - If true, hidden text is displayed.
|
ShowHoriRuler | boolean - If true, the horizontal ruler is displayed.
|
ShowHoriScrollBar | boolean - If true, the horizontal scroll bar is displayed.
|
ShowIndexMarkBackground | boolean - If true , index marks are displayed with gray background.
|
ShowParaBreaks | boolean - If true , paragraph breaks are visible.
|
ShowProtectedSpaces | boolean - If true, protected spaces (hard spaces) are displayed with gray background.
|
ShowSoftHyphens | boolean - If true, soft hyphens are displayed with gray background.
|
ShowSpaces | boolean - If true, spaces are displayed with dots.
|
ShowTableBoundaries | boolean - If true, table boundaries are displayed.
|
ShowTables | boolean - If true, tables are visible.
|
ShowTabstops | boolean - If true, tab stops are visible.
|
ShowTextBoundaries | boolean - If true, text boundaries are displayed.
|
ShowTextFieldBackground | boolean - If true, text fields are displayed with gray background.
|
ShowVertRuler | boolean - If true, the vertical ruler is displayed.
|
ShowVertScrollBar | boolean - If true, the vertical scroll bar is displayed.
|
SmoothScrolling | boolean - If true, smooth scrolling is active.
|
SolidMarkHandles | boolean - If true, handles of drawing objects are visible.
|
ZoomType | short - defines the zoom type for the document as defined in com.sun.star.view.DocumentZoomType
|
ZoomValue | short - defines the zoom value to use, the value is given as percentage. Valid only if the property ZoomType is set to BY_VALUE.
|
In StarOffice 6.0 and OpenOffice.org 1.0 you can only influence the zoom factor by setting the ZoomType
to BY_VALUE
and adjusting ZoomValue
explicitly. The other zoom types have no effect.
TextViewCursor
The text controller has a visible cursor that is used in the GUI. Get the com.sun.star.text.TextViewCursor by calling getTextViewCursor()
at the com.sun.star.text.XTextViewCursorSupplier interface of the current text document controller.
It supports the following cursor capabilities that depend on having the necessary information about the current layout state, therefore it is not supported by the model cursor.
boolean jumpToFirstPage() boolean jumpToLastPage() boolean jumpToPage( [in] long pageNo) long getPage() boolean jumpToNextPage() boolean jumpToPreviousPage() boolean jumpToEndOfPage() boolean jumpToStartOfPage()
com.sun.star.view.XScreenCursor
boolean screenDown() boolean screenUp()
boolean goDown( [in] long lines, [in] boolean bExpand) boolean goUp( [in] long lines, [in] boolean bExpand) boolean isAtStartOfLine() boolean isAtEndOfLine() void gotoEndOfLine( [in] boolean bExpand) void gotoStartOfLine( [in] boolean bExpand)
boolean goLeft( [in] long characters, [in] boolean bExpand) boolean goRight( [in] long characters, [in] boolean bExpand) boolean goDown( [in] long characters, [in] boolean bExpand) boolean goUp( [in] long characters, [in] boolean bExpand)
Additionally the interface com.sun.star.beans.XPropertySet is supported.
Currently, the view cursor does not have the capabilities as the document cursor does. Therefore, it is necessary to create a document cursor to have access to the full text cursor functionality. The method createTextCursorByRange()
is used:
XText xCrsrText = xViewCursor.getText();
// Create a TextCursor over the view cursor's contents
XTextCursor xDocumentCursor = xViewText.createTextCursorByRange(xViewCursor.getStart());
xDocumentCursor.gotoRange(xViewCursor.getEnd(), true);